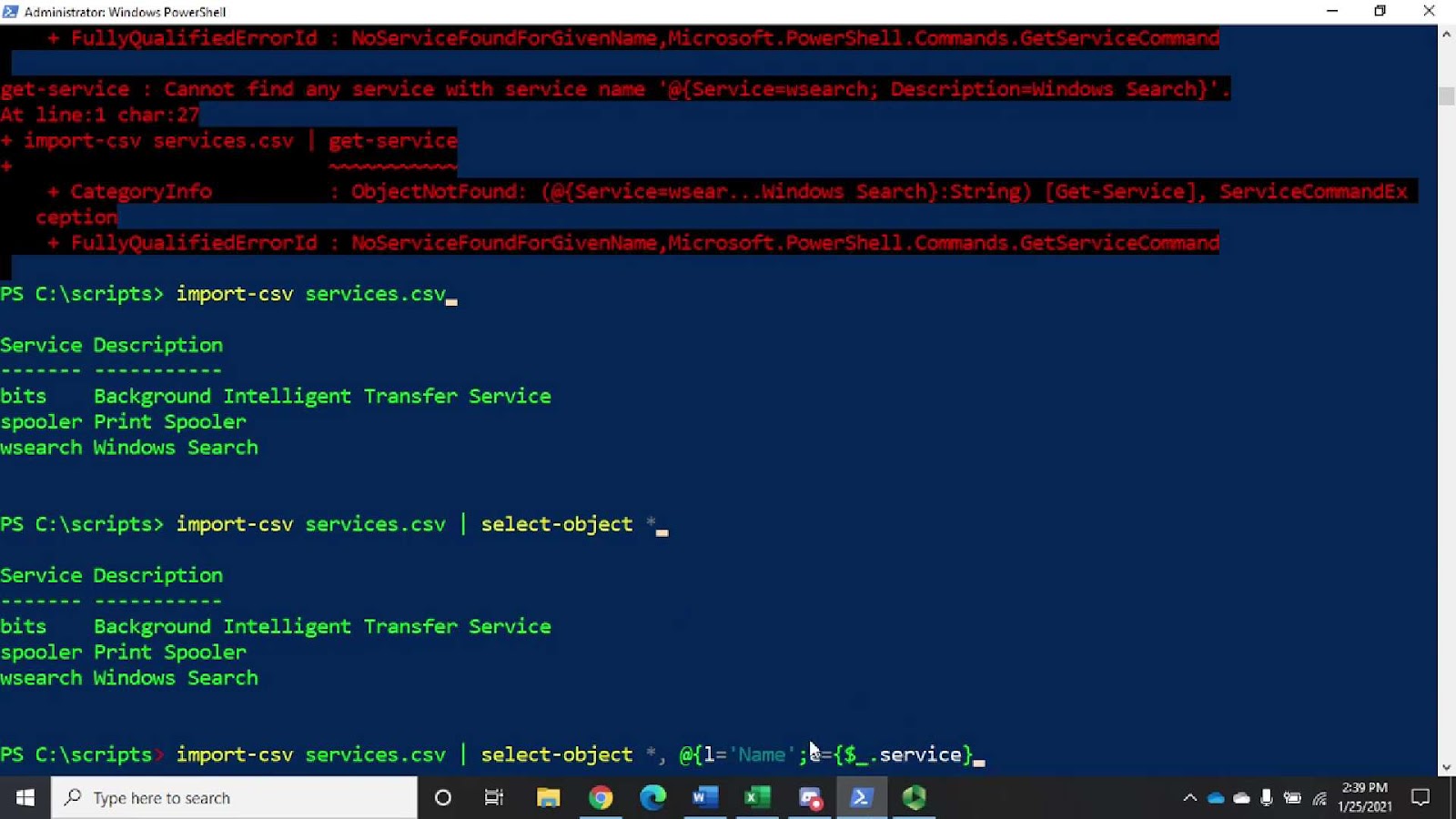
Unlocking the Potential of PowerShell’s Input Pipelines
In the realm of system administration and automation, PowerShell emerges as the preferred and potent tool among IT professionals. Its adaptability and robust scripting capabilities render it an indispensable asset for the orchestration and configuration of Windows environments. At the core of PowerShell’s prowess resides the pivotal concept of the pipeline—a dynamic mechanism that facilitates the seamless flow of both data and commands.
Within the confines of this article, we shall embark on a comprehensive exploration of the fundamental topic of PowerShell pipeline input. This journey will illuminate how this feature empowers users to execute intricate tasks with a touch of grace and unparalleled efficiency. Whether you find yourself a seasoned veteran or a newcomer seeking to unlock the boundless potential of this commanding scripting language, grasping the pipeline’s handling of input is an imperative cornerstone in your quest to master the art of automation.
As we delve deeper into the intricacies of PowerShell’s pipeline input, prepare to embark on a voyage of discovery. By the time we conclude, you will have unveiled the enigmatic facets of this feature and witnessed how it possesses the transformative capability to elevate your scripting prowess to unprecedented heights. Join us in this enlightening journey as we decode the mysteries surrounding PowerShell’s pipeline input, uncovering its capacity to transcend boundaries and amplify your scripting skills.
Comprehending the PowerShell Pipeline
In the PowerShell scripting environment, a key component is the pipeline. The pipeline employs the operator ‘|’, allowing the conduction of commands in a sequence where the output of one command can be used as the input for the following command. This tool enables the seamless linking of multiple commands, tailored to accomplish the desired task efficiently.
Structural Essence of the Pipeline
The foundational structure of the pipeline can be envisioned as follows:
Command1 (output)
→
∣
Command2 (output)
→
∣
Command3
Command1 (output)
∣
Command2 (output)
∣
Command3
Practical Implementation of the Pipeline
To commence exploring the pipeline’s utility, consider the command Get-Process. This command unveils a list displaying all the processes currently running, providing varied information pertaining to each process, enabling users to assess system performance and monitor activities.
Refinement and Sorting
Suppose one wishes to focus solely on the first ten processes and desires them sorted based on CPU usage. For this refinement, the following command sequence can be used:
Get-Process
∣
Sort-Object CPU -Descending
∣
Select-Object -First 10
Get-Process∣Sort-Object CPU -Descending∣Select-Object -First 10
Here, Get-Process is linked to Sort-Object through the pipeline, allowing the sorting of processes based on CPU usage in descending order, and subsequently piped to Select-Object to narrow down the results to the first ten.
To explore the opposite end of the spectrum, one can simply alter the final segment of the command to -Last 10 to visualize the last ten results:
Get-Process
∣
Sort-Object CPU -Descending
∣
Select-Object -Last 10
Get-Process∣Sort-Object CPU -Descending∣Select-Object -Last 10
Visualization Enhancement
For users aspiring for a more visually distinctive representation, the concluded command can be further piped to Out-GridView. This presents the information in a grid view, offering a structured and clear presentation of the results:
Get-Process
∣
Sort-Object CPU -Descending
∣
Select-Object -Last 10
∣
Out-GridView
Get-Process∣Sort-Object CPU -Descending∣Select-Object -Last 10∣Out-GridView
Accepting Pipeline Input in PowerShell Functions
Embracing the incorporation of pipeline input within your PowerShell scripts can substantially elevate their adaptability and utility. This capability empowers you to effortlessly manipulate data originating from diverse origins, enabling efficient execution of operations. Within the following discourse, we shall delve into the art of harnessing pipeline input, employing parameter attributes, and delineating the multifaceted segments within a PowerShell function.
Enhancing Pipeline Input with Parameter Attributes
Within the domain of PowerShell’s pipeline, there exists an elegant approach to seamlessly integrate input data – the incorporation of parameter attributes within the [cmdletbinding()] section of your script. This pivotal juncture presents two widely embraced parameter attributes at your disposal:
Embracing ValueFromPipeline: This attribute readily accepts input values directly streamed through the pipeline.
Unleashing the Potential of ValueFromPipeline
The ValueFromPipeline parameter attribute serves as your conduit to tap into the formidable capabilities of the PowerShell pipeline. It gracefully captures all values transmitted via the pipeline. To illustrate its functionality, consider the following illustrative scenario:
function Write-PipeLineInfoValue {
[cmdletbinding()]
param(
[parameter(
Mandatory = $true,
ValueFromPipeline = $true)]
$pipelineInput
)
Begin {
# Code in the Begin block runs once at the start and is suitable for setting up variables.
Write-Host `n"The begin {} block runs once at the start, and is good for setting up variables."
Write-Host "-------------------------------------------------------------------------------"
}
Process {
# Code in the Process block handles pipeline input.
# It's advisable to process each element individually in a ForEach loop.
ForEach ($inputItem in $pipelineInput) {
Write-Host "Processing [$($inputItem.Name)] information"
if ($inputItem.Path) {
Write-Host "Path: $($inputItem.Path)`n"
} else {
Write-Host "No path found!"`n -ForegroundColor Red
}
}
}
End {
# Code in the End block runs once at the end and is perfect for cleanup tasks.
Write-Host "-------------------------------------------------------------------------------"
Write-Host "The end {} block runs once at the end, and is good for cleanup tasks."`n
}
}
Get-Process | Select-Object -First 10 | Write-PipeLineInfoValue
Results of Pipeline Input Processing
When you accept pipeline input, it is primarily handled within the Process {} block of your function. However, you can also utilize the Begin {} and End {} blocks for additional control:
The Begin {} block: This block runs once when the function is invoked. It’s where you can set up variables and perform initial setup tasks.
Begin {
Write-Host `n"The begin {} block runs once at the start, and is good for setting up variables."
Write-Host "-------------------------------------------------------------------------------"
}
The Process {} block: This is where the pipeline input is processed. It’s advisable to handle each pipeline element individually using a ForEach loop.
Process {
ForEach ($inputItem in $pipelineInput) {
# Processing logic for each input item
}
}
The End {} block: Code within this block runs after all pipeline elements are processed. It's a suitable place for cleanup tasks and finalization.
powershell
Copy code
End {
Write-Host "-------------------------------------------------------------------------------"
Write-Host "The end {} block runs once at the end, and is good for cleanup tasks."`n
}
Grasping the intricacies of these building blocks and employing the ValueFromPipeline parameter attribute enhances the adaptability and performance of your PowerShell functions when dealing with pipeline input.
Parameter Attribute – ValueFromPipelineByPropertyName
In the realm of PowerShell, a profound grasp of parameter attributes becomes imperative to unlock the true capabilities of your scripts and functions. Among these attributes, there emerges a standout known as “ValueFromPipelineByPropertyName.” This particular attribute distinguishes itself with an intriguing twist—it discriminately embraces input from the pipeline by scrutinizing property name correlations. Let’s embark on a deeper exploration of this captivating attribute and discern how it can elevate your prowess in the art of PowerShell scripting.
How it Works
When you designate a parameter with ValueFromPipelineByPropertyName, you’re essentially telling PowerShell to filter incoming pipeline objects, considering only those whose property names align with the parameter’s name. For instance, let’s take a look at this illustrative example:
function Write-PipeLineInfoPropertyName {
[cmdletbinding()]
param(
[parameter(
Mandatory = $true,
ValueFromPipelineByPropertyName = $true)]
[string[]]
$Name
)
Begin {
Write-Host `n"The begin {} block runs once at the start, and is good for setting up variables."
Write-Host "-------------------------------------------------------------------------------"
}
Process {
ForEach ($input in $name) {
Write-Host "Value of input's Name property: [$($input)]"
}
}
End {
Write-Host "-------------------------------------------------------------------------------"
Write-Host "The end {} block runs once at the end, and is good for cleanup tasks."`n
}
}
Practical Application
Now that we understand how this parameter attribute operates, let’s explore some practical applications:
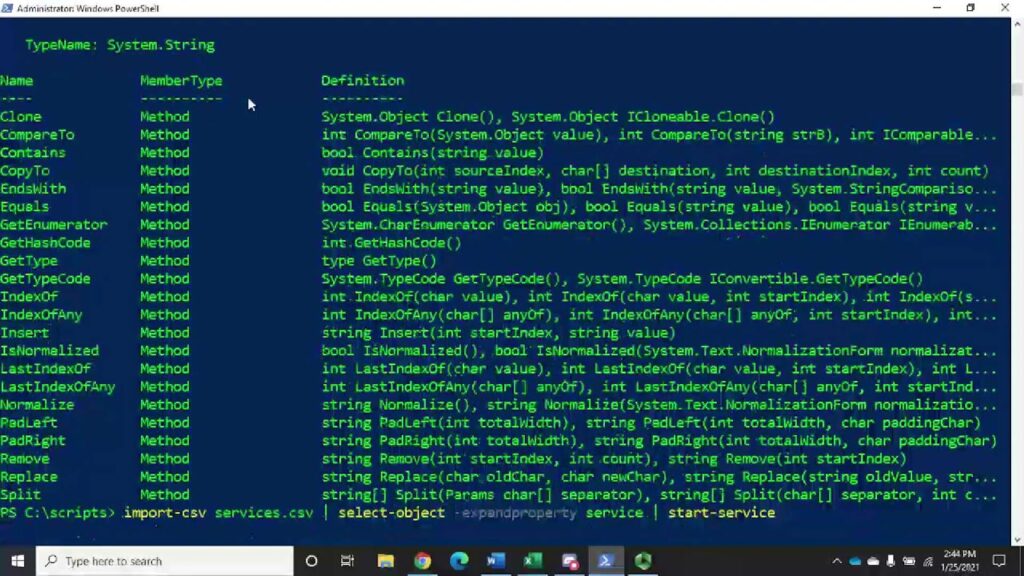
1. Computer Management
Imagine you need to perform actions on a list of specific computers. By piping this list to a function that utilizes ValueFromPipelineByPropertyName, you can effortlessly execute actions on each computer, simplifying complex tasks like remote management and administration.
2. Logging Functions
If you have a logging function that can process bulk messages or commands, you can easily pipe these commands to it. This allows for efficient log file creation and management, streamlining the monitoring and troubleshooting process.
3. Flexibility and Choice
PowerShell offers multiple ways to accomplish tasks, and piping commands together is just one approach. Depending on your specific needs and preferences, you can decide whether using ValueFromPipelineByPropertyName is the most elegant solution or if there’s a better alternative for your particular scenario.
Conclusion
To sum up, the essence of the PowerShell scripting language hinges on the foundational and formidable concept of the PowerShell pipeline input. This concept serves as the central axis, enabling a fluid and effortless exchange of data between cmdlets, thereby facilitating the creation of sophisticated and highly efficient automation scripts with remarkable simplicity. By granting the privilege of utilizing the output from one cmdlet as the raw material for another, PowerShell bestows upon users the capability to execute an extensive array of tasks, spanning from elementary data manipulation to intricate system administration.