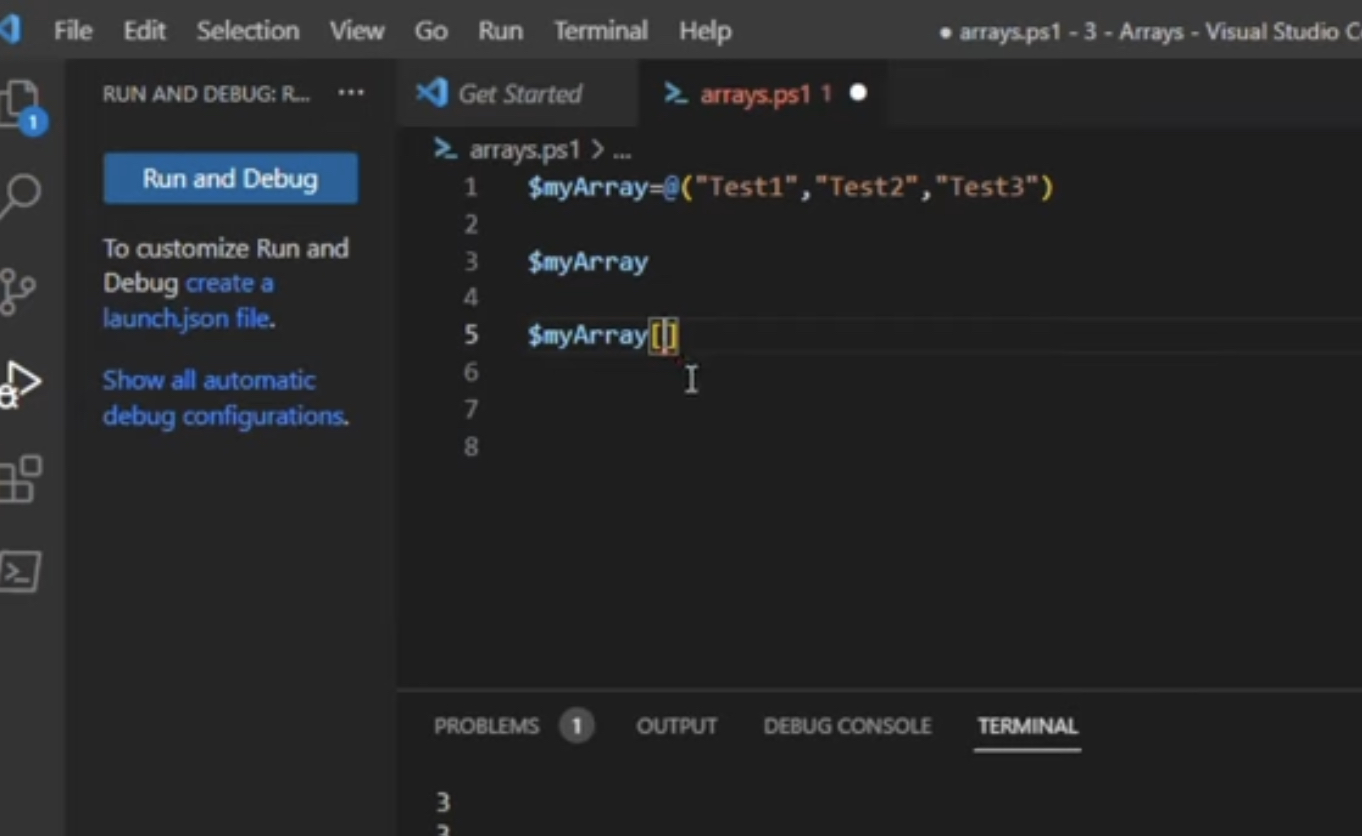
PowerShell Array of Arrays: Efficient Data Management
In the realm of data structures, an array emerges as a vessel crafted for the purpose of containing a multitude of items, be they of uniform or diverse natures.
Creating Arrays
To give birth to an array, one can adopt various methods. The first involves the usage of the `@()` syntax:
```powershell
$values = @("One", "Two", "Three", "Four", "Five")
```
Here, we define an array named `$values` with the elements “One,” “Two,” “Three,” “Four,” and “Five.”
```powershell
$values
$values.GetType()
```
Resulting in:
```
One
Two
Three
Four
Five
IsPublic IsSerial Name BaseType
True True Object[] System.Array
```
Another approach entails setting an array with comma-separated values:
```powershell
$values = "Six", "Seven", "Eight", "Nine", "10"
```
Resulting in:
```
Six
Seven
Eight
Nine
10
```
You may also create an array with a specified type, like so:
```powershell
[int[]]$values = 6, 7, 8, 9, 10
```
Resulting in:
```
6
7
8
9
10
```
Or opt for the `[array]` type:
```powershell
[array]$values = 11, 12, 13, 14, 15
```
Resulting in:
```
11
12
13
14
15
```
Adding and Modifying Array Elements
Once an array is in existence, items can be added or altered. For instance:
```powershell
$values = @("One", "Two", "Three")
```
To ascertain the number of items within the array:
```powershell
Write-Host "Items in array $($values.Count)"
```
To append an item:
```powershell
$values += "Four"
```
And again, to inspect the count:
```powershell
Write-Host "Items in array $($values.Count)"
```
To alter an item by its index:
```powershell
$values[0] = "Five"
```
Resulting in:
```
One
Two
Three
Items in array 3
One
Two
Three
Four
Items in array 4
Five
Two
Three
Four
```
Accessing Arrays
To access the treasures stored within an array, one must employ the index, commencing with 0:
```powershell
[array]$values = 1, 2, 3, 4, 5
```
To unveil the third item:
```powershell
Write-Host "Item at index 2: $($values[2])"
```
Resulting in:
```
Item at index 2: 3
```
Navigating Arrays via Loops
The index approach remains relevant when traversing arrays through loops. An array of names, for instance:
```powershell
$nameArray = @("Erik", "Penny", "Randy", "Sandy", "Toby", "Uma", "Vicky", "Will", "Xavier", "Yvette", "Zach")
```
Can be explored using a `for` loop:
```powershell
for ($i = 0; $i -lt $nameArray.Length; $i++) {
Write-Host $nameArray[$i]
}
```
Resulting in:
```
Erik
Penny
Randy
Sandy
Toby
Uma
Vicky
Will
Xavier
Yvette
Zach
```
To wrap up
In conclusion, arrays stand as versatile data structures capable of storing collections of items, whether they are of the same or different types. This exploration has illuminated various methods of creating arrays, from using the `@()` syntax to specifying types, along with techniques for adding, modifying, and accessing their elements. Additionally, the power of loops in navigating arrays has been showcased. With this understanding, one can harness the potential of arrays to organize and manipulate data effectively in PowerShell.