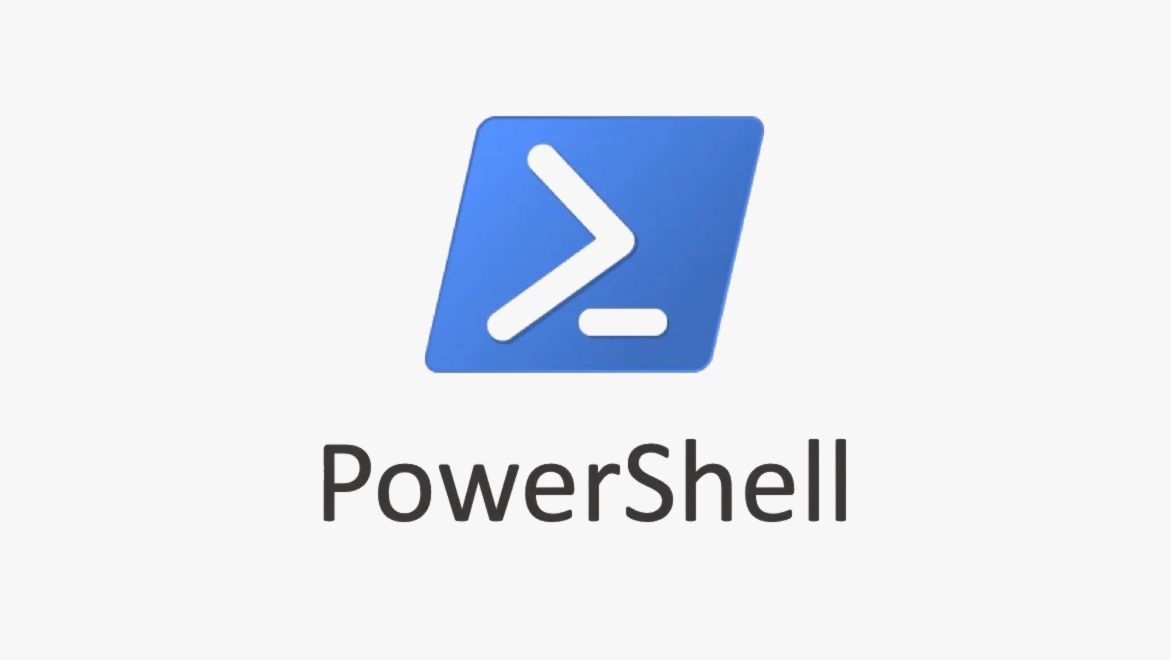
Mastering Error Handling in Powershell
When developing scripts in PowerShell, it is crucial to implement effective error-handling strategies to manage and resolve issues that may arise during execution.
This article delves deep into PowerShell error handling, offering a rich understanding of exceptions, their handling, and the methodology to develop resilient scripts. It discusses strategies to identify, catch, and manage errors, ensuring smooth execution and avoiding unexpected halts in script runs.
Understanding PowerShell Error Handling
The saying, “Anything that can go wrong, will go wrong,” resonates deeply with developers and programmers. Crafting flawless scripts often seems like an attainable goal, yet unforeseen variables can lead to malfunctions.
This article examines the integral process of anticipating and managing errors—error handling. We will dissect a concise program and highlight essential lines and functions that elucidate how to detect and identify errors instead of letting your scripts run with invalid inputs.
Defining a PowerShell Exception
In PowerShell, an exception refers to an error occurring during script execution which necessitates handling. By default, PowerShell attempts to manage errors independently; however, some errors, known as exceptions, surpass its capability to do so.
The occurrence of an exception is termed as “thrown,” and managing a thrown exception is denoted as “catching” it, directing the script on the subsequent course of action. If an exception is not caught, it results in script termination.
Importance of PowerShell Error Handling
Error handling is paramount in PowerShell as it distinguishes an ordinary script from a highly reliable one by its response to anticipated errors. Efficient error handling not only informs the user about what’s wrong, akin to a patient describing symptoms to their doctor but also prevents wastage of time and resources by halting a process destined to fail due to an exception.
It is imperative to discern that error handling is not synonymous with incorrect scripting; it’s about identifying external variables beyond the control of your program.
When Is Error Handling Crucial?
Effective error handling is a defining feature of proficient scripts. The proactive anticipation and response to errors significantly improve the performance and reliability of scripts. For a developer, incorporating error handling is essential as it aids in multiple ways.
It prevents the continuation of futile processes that are destined for failure, thus conserving time and resources and elevating the quality of the program.
Techniques to Code PowerShell Error Handling
Coding error handling in PowerShell involves three pivotal steps: identification of potential errors, encapsulating the command within a ‘try’ block, and defining the remedial actions within a ‘catch’ block in case of an error occurrence. It is generally optimal to focus error handling on individual commands for maximum efficacy.
Most PowerShell commands necessitate a slight modification within the ‘try’ block. A parameter, ErrorAction, needs to be added to the function and set to “Stop”. This can sometimes be abbreviated as -EA. In some scenarios, depending on the type of error, different actions may be required. For such cases, the error can be captured in an -ErrorVariable, typically abbreviated as -EV.
Detailed Script Analysis
Examining the following script will help identify where errors might occur.
param (
$computername = 'localhost'
)
$os = Get-WmiObject -Class Win32_OperatingSystem -ComputerName $computername
$system = Get-WmiObject -Class Win32_ComputerSystem -ComputerName $computername
In this scenario, errors are likely to occur in the lines where data from an external source is being accessed. Potential errors include unavailability of the computer when queried or lack of permission to execute the query. Addressing such errors is crucial for the successful execution of the script.
Handling Errors in PowerShell Scripts
When it comes to scripting, especially in PowerShell, unexpected errors can arise. It’s not just about identifying these errors; the real challenge lies in deciding how to address them. Consider a scenario where you wish to document the name of a computer that doesn’t respond. One approach is to utilize the variable $computername and append it to an existing log file.
try {
$os = Get-WmiObject -Class Win32_OperatingSystem -ComputerName $computername -ErrorAction Stop -ErrorVariable x
} catch {
}
It might also be beneficial to display a warning message to enhance user awareness. This warning can be a standardized message that indicates the problem.
try {
$os = Get-WmiObject -Class Win32_OperatingSystem -ComputerName $computername -ErrorAction Stop -ErrorVariable x
} catch {
$computername | Out-File -Path "c:\errors.txt" -Append
}
Make sure there’s a space following the $computername variable. By enclosing the message in double quotes, it allows for variables to display their corresponding values.
Effective Error Handling in PowerShell
The essence of effective error management in PowerShell is introducing structured logic into your code. Exceptional error handling not only detects errors but also predicts potential consequences.
Let’s evaluate the enhancements made:
try {
$os = Get-WmiObject -Class Win32_OperatingSystem -ComputerName $computername -ErrorAction Stop -ErrorVariable x
} catch {
$computername | Out-File -Path "c:\errors.txt" -Append
Write-Warning "Encountered an error with $computername : $x"
}
$systemInfo = Get-WmiObject -Class Win32_ComputerSystem -ComputerName $computername
$properties = @{
'ComputerName' = $computername;
'OSVersion' = $os.caption;
'TotalRAM' = $systemInfo.TotalPhysicalMemory;
'Manufacturer' = $systemInfo.Manufacturer
}
$obj = New-Object -TypeName PSObject -Property $properties
Write-Output $obj
However, what if the computer doesn’t reply to the initial query? This is where introducing conditions can be crucial. If the first request fails, there’s a good chance the subsequent ones will too.
try {
$everything_is_fine = $true
$os = Get-WmiObject -Class Win32_OperatingSystem -ComputerName $computername -ErrorAction Stop -ErrorVariable x
} catch {
$computername | Out-File -Path "c:\errors.txt" -Append
Write-Warning "Encountered an error with $computername : $x"
$everything_is_fine = $false
}
if ($everything_is_fine) {
$systemInfo = Get-WmiObject -Class Win32_ComputerSystem -ComputerName $computername
$properties = @{
'ComputerName' = $computername;
'OSVersion' = $os.caption;
'TotalRAM' = $systemInfo.TotalPhysicalMemory;
'Manufacturer' = $systemInfo.Manufacturer
}
$obj = New-Object -TypeName PSObject -Property $properties
Write-Output $obj
}
In this construct, if the initial function encounters an issue, the instructions inside the catch block get executed. If this occurs, the $everything_is_fine variable is set to false, preventing the execution of subsequent lines.
This example, though simple, highlights a strategy to handle not just the first exception, but also offers a pathway for subsequent functions. By understanding and employing such methods, one can ensure that their PowerShell scripts are robust and resilient against unforeseen errors.
Assessing Error Handling Strategies in PowerShell
When designing automation scripts via PowerShell, rigorous testing of code is pivotal. It is crucial to assess not only the success states but also the failure states of a program. Testing is easily achievable with local devices, and with the detailed examples provided, professionals can verify the operation of localhost servers, ensuring their discoverability on the network. This typically yields successful results.
However, the true prowess of error management is witnessed when failures are scrutinized. Testing errors can be facilitated by manipulating parameters, for instance, modifying ‘localhost’ to a non-existent server, denoted as ‘NOTONLINE’ in the examples. When executed, this triggers errors, revealing comprehensive exception details such as “The RPC server is unavailable.”
Beyond just on-screen revelations, errors can also be recorded in log files, allowing for precise documentation of encountered issues. This approach underscores the importance of ‘-ErrorAction’, a crucial component in PowerShell, which instructs the command to halt and throw a trappable exception upon encountering a problem, ensuring that the try-catch block functions effectively.
Understanding the Repercussions of Removing ErrorAction
Eliminating ‘-EA Stop’ from the code alters the program significantly. Running the manipulated code, particularly with a non-existent server name, yields less manageable errors and prolongs the Windows Management Instrumentation timeout period. Such errors are not mere warnings; they are uncaught exceptions emanating directly from the command, rendering the second command’s output unintelligible due to faulty input.
This emphasizes the importance of trapping each exception as a warning to prevent wastage of time, energy, and resources, and to pinpoint the location and nature of errors more effectively.
By imposing stops upon encountering errors, users can address the causative issues promptly, leveraging ‘-ErrorAction Stop’ as a pivotal tool for optimizing error management in PowerShell.
Importance of Efficient Error Management
Inadequate error management can lead PowerShell to execute commands inaccurately, utilizing corrupted data derived from successive errors. However, by instructing the system to halt during errors, users can rectify the causative factors swiftly and efficiently.
To elevate your proficiency in PowerShell, seeking advanced training is highly recommended. While this article touches upon the essential aspects of error management in PowerShell, extensive training sessions, encompassing extensive hours of instruction and multiple tutorial videos, can provide in-depth insights into scripts and automation.
Comprehensive Strategies for Enhanced Error Handling
- Rigorous Testing: Regularly test both the success and failure states of the program to identify potential errors and rectify them in a timely manner;
- Log File Documentation: Utilize log files to record and analyze errors systematically for effective resolution;
- Utilize ErrorAction: Employ ‘-ErrorAction Stop’ to ensure the try-catch block functions effectively and to manage errors efficiently;
- Enhanced Training: Seek advanced training sessions for comprehensive insights into scripts, automation, and optimal error management strategies.
Conclusion
In conclusion, efficient error handling in PowerShell is crucial for the development of robust and reliable automation scripts. By implementing comprehensive testing, utilizing effective error management tools like ‘-ErrorAction’, and seeking advanced training, professionals can significantly enhance their scripting capabilities. Effective error handling not only saves time, energy, and resources but also ensures the delivery of accurate and reliable automation solutions.
Balancing the exploration between success states and failure states, leveraging log files for systematic documentation and analysis, and embracing advanced learning opportunities are pivotal steps toward mastering sophisticated scripting and automation tasks in PowerShell.