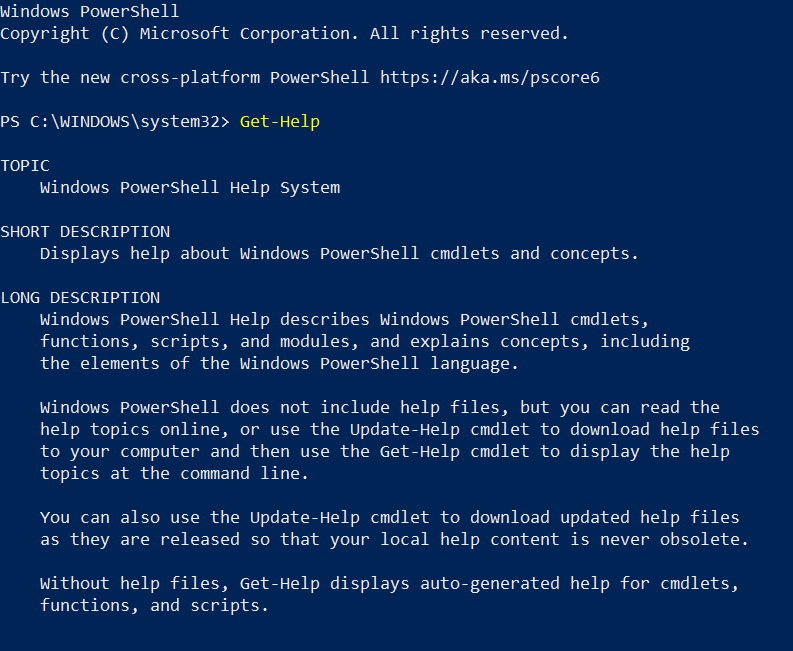
PowerShell for Efficient Automation: Mastering Key Commands
In the world of scripting, both programmers and non-programmers find PowerShell to be a valuable tool. Its ability to automate tasks, whether simple or complex, makes it indispensable for businesses, daily routines, and even recreational purposes. One particularly challenging aspect of automation lies in crafting loops for scripts. Loops are the key to streamlining repetitive tasks that consume precious time. This tutorial delves into various loop types in PowerShell, including the For loop, ForEach-Object loop, and the While, Do-While, and Do-Until loops.
What Sets PowerShell Apart?
PowerShell, an open-source scripting language, serves as a bridge for tech professionals who may not have a background in software programming. Its dual nature encompasses a command-line shell akin to the Windows command prompt (known as “cmd.exe”) and a powerful scripting language capable of automating almost any task. The language’s genesis dates back to 2002 when Jeffrey Snover, then at Microsoft, sought to rival Linux’s command-line capabilities. Thus, PowerShell was born and eventually became the default installation on all Windows platforms starting with Windows 7.
Like other shells, PowerShell boasts binary commands for executing actions, from reading files to pinging computers and deleting registry keys. It also incorporates cmdlets, compiled binaries that empower users to build essential tools for their scripts. Notably, PowerShell adheres to a Verb-Noun syntax, where command names begin with verbs, feature a dash in the middle, and conclude with a noun. This syntax is designed to convey the action performed by the cmdlet. For instance, “Copy-Item” copies files, and “Get-Content” retrieves text from a file. If you’re uncertain about the verb to use, “Get-Verb” provides a list of accepted verbs. While PowerShell doesn’t have approved nouns, “Get-Command” helps identify available objects considered nouns.
Harnessing the Power of Loops in PowerShell
PowerShell loops, at their core, automate tasks by repeating a set of commands a predetermined number of times. These loops excel in handling consistent actions over a specific duration or a designated number of records, simplifying script development. PowerShell offers an array of cmdlets, particularly those starting with “Get,” which return objects containing extensive data.
- The ForEach-Object loop, for instance, is an elegant choice when iterating through objects, providing a streamlined way to process each member efficiently. Its flexibility extends to pre-loop and post-loop actions through the -Begin and -End parameters, allowing for advanced scripting control;
- On the other hand, the PowerShell For loop shines in scenarios where a specific number of iterations is required. It simplifies tasks like generating multiplication tables or traversing arrays, contributing to cleaner and more concise code;
- The While, Do-While, and Do-Until loops cater to situations where looping should continue as long as a certain condition is met or until it becomes true. These conditional loops provide the necessary tools for dynamic script execution, adapting to changing circumstances;
- However, the art of choosing the right loop is not just about functionality; it’s about optimizing script performance and enhancing code readability. Striking this balance ensures that PowerShell scripts run efficiently and remain comprehensible for both the scriptwriter and potential collaborators.
In essence, PowerShell’s array of loop options empowers users to approach automation with tailored solutions, making it a valuable tool for tasks ranging from routine system maintenance to complex data processing. Understanding and harnessing these loops are fundamental steps towards mastering PowerShell’s capabilities for automation and scripting.
The ForEach-Object Loop
The ForEach-Object cmdlet often emerges as the optimal choice for looping through objects. It simplifies the process by necessitating only two components: the object to be looped through and a script block containing the commands to execute on each object member. Users have the flexibility to define these parameters in multiple ways, either by specifying the object with the -InputObject parameter and the script block with the -Process parameter names, or by employing the piping feature, where the object is piped into ForEach-Object with the script block as the first parameter. For practical illustration, consider the scenario of looping through a user’s Documents folder. In this case, two methods demonstrate the usage of ForEach-Object effectively:
```powershell
$myDocuments = Get-ChildItem $env:USERPROFILE\Documents -File
$myDocuments | ForEach-Object {$_.FullName}
```
This code first retrieves the files in the Documents folder and assigns them to the $myDocuments variable. Then, it pipes this variable into ForEach-Object, executing the script block to retrieve the full names of the files. The simplicity and adaptability of ForEach-Object make it an invaluable tool when dealing with objects, further enhancing PowerShell’s reputation as a versatile scripting language.
“`
In some cases, it proves advantageous to perform actions before or after the loop. The -Begin and -End parameters facilitate the execution of script blocks before and after the -Process script block, allowing variable modifications at these points. ForEach-Object boasts two aliases, “ForEach” and “%,” and supports shorthand syntax, introduced in PowerShell 3.0, as seen in these equivalent examples:
```powershell
Get-WMIObject Win32_LogicalDisk | ForEach-Object {$_.FreeSpace}
Get-WMIObject Win32_LogicalDisk | ForEach {$_.FreeSpace}
Get-WMIObject Win32_LogicalDisk | % FreeSpace
```
Unveiling the PowerShell For Loop
For loops are designed for iterating through a sequence of commands a specified number of times, whether to traverse an array or simply repeat a block of code as needed. Constructed with an initial variable value, a termination condition, and an action to perform on the variable within the loop, the For loop offers versatility. Here’s a basic example generating a multiplication table:
```powershell
For ($i=0; $i -le 10; $i++) {
"10 * $i = " + (10 * $i)
}
```
For loops excel at stepping through array values, allowing manipulation by specifying the incremented variable inside square brackets immediately following the variable name. This code demonstrates how to iterate through an array of colors:
```powershell
$colors = @("Red", "Orange", "Yellow", "Green", "Blue", "Indigo", "Violet")
For ($i=0; $i -lt $colors.Length; $i++) {
colors[$i]
}
```
Mastering While, Do-While, and Do-Until Loops
PowerShell supports a third type of loop that operates based on a condition—either as long as the condition holds true or until it becomes true. While and Do-While loops share a commonality; both execute an action while the condition remains true, differing only in syntax. On the other hand, Do-Until loops maintain a similar structure to Do-While loops but cease execution once the condition is met.
Both Do-While and Do-Until loops begin with the “Do” keyword, followed by a script block, the condition keyword (“While” or “Until”), and the condition. For instance, these two loops perform identically, differing only in the condition:
```powershell
$i=1
Do {
$i
$i++
}
While ($i -le 10)
$i=1
Do {
$i
$i++
}
Until ($i -gt 10)
```
While loops mirror the functionality of Do-While loops, with a slight syntax adjustment. They employ only the “While” keyword, followed by the condition and the script block. This loop operates similarly to the previous examples, using the same condition as the Do-While loop:
```powershell
$i=1
While ($i -le 10)
{
$i
$i++
}
```
All three loop types—Do-While, Do-Until, and While—can be employed for indefinite looping by setting conditions to $true or $false.
In situations where exiting a loop prematurely is necessary, the “Break” keyword provides an escape route. The following example demonstrates this functionality through an infinite loop, using the “Break” keyword to exit at the appropriate moment:
```powershell
$i=1
While ($true)
{
$i
$i++
if ($i -gt 10) {
Break
}
}
```
Contributor: Sean Peek
Understanding PowerShell and its diverse array of loops opens the door to efficient scripting and task automation. Whether you’re a seasoned programmer or a newcomer to the world of automation, PowerShell’s versatility and loop options offer a powerful toolkit for streamlining your work.
- In conclusion, PowerShell stands as a versatile scripting language, bridging the gap between programmers and non-programmers in their quest for efficient automation. Its dual nature, encompassing a command-line shell and a powerful scripting language, empowers users to streamline their daily tasks, making it a valuable asset for businesses, professionals, and enthusiasts alike;
- The realm of loops in PowerShell further solidifies its utility. These loops, from the straightforward For loop to the flexible ForEach-Object loop and the conditional While, Do-While, and Do-Until loops, provide the means to automate tasks efficiently. Whether you need to repeat a set of commands a specific number of times, traverse an array, or operate based on conditions, PowerShell’s loop options cater to various needs;
- Additionally, PowerShell’s Verb-Noun syntax and extensive library of cmdlets and functions make it a robust choice for scripting. This structured approach to command naming, combined with its adaptability, enables users to craft scripts that can automate a wide range of tasks, from file operations to system management.
In essence, mastering PowerShell and its diverse loop capabilities empowers individuals and organizations to save time, enhance productivity, and unlock the full potential of automation. Whether you’re a newcomer exploring the world of scripting or a seasoned professional seeking efficiency, PowerShell remains a formidable tool in your arsenal, bridging the gap between simplicity and power in the world of automation.