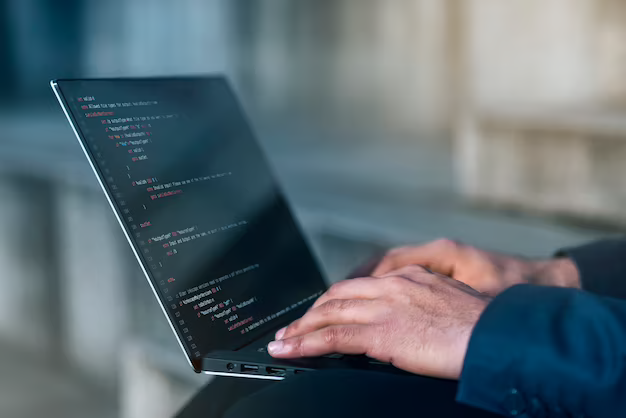
PowerShell ValidateSet: Dive into Parameter Validation
In the realm of PowerShell scripting, one of the gems that often goes unnoticed is ValidateSet. This powerful feature falls under advanced parameters and allows you to restrict input to a predefined set of values. It even provides autocompletion for these options, simplifying user interaction. But why should you bother with ValidateSet, and how can it elevate your scripting game? Let’s dive into this essential tool and explore its myriad applications.
Why Use ValidateSet?
Imagine you’re crafting a PowerShell script, and you want to ensure that a particular parameter accepts only a specific set of values. This is where ValidateSet shines. It not only enforces input constraints but also enhances user experience by offering auto-suggestions as users tab through the options.
Here are scenarios where ValidateSet proves invaluable:
- Active Directory Operations: When working with scripts that interact with Active Directory, you often want to limit the choices for certain parameters, such as user roles or group names;
- API Integration: Scripts interfacing with APIs frequently require parameters that accept a predefined set of values, ensuring compatibility with the external service;
- Web Parsing: In web scraping or parsing scripts, you might want to restrict options for parameters like data sources, output formats, or specific parsing modes;
- Enhanced Script Clarity: By offloading logic to parameter validation, you can streamline your script, making it more readable and easier to maintain. Additionally, it simplifies error handling by providing predefined validation checks.
Using ValidateSet
Implementing ValidateSet is a breeze. You need to add a simple line above your parameter declaration like so:
[ValidateSet(‘Option1’, ‘Option2’, ‘Option3’)] |
This declaration ensures that the input for the associated parameter is limited to the provided options. Let’s illustrate how it works with a straightforward function:
function Write-Color { [cmdletbinding()] param( [Parameter(Mandatory)] [ValidateSet(‘Green’, ‘Blue’, ‘Red’)] [string] $color, $message ) Write-Host $message -ForegroundColor $color} |
In this example, the $color parameter is constrained to accept only three values: ‘Green,’ ‘Blue,’ or ‘Red.’
Here’s how you can use this function:
Write-Color -color Blue -Message “Validate: Blue”Write-Color -color Red -Message “Validate: Red”Write-Color -color Green -Message “Validate: Green” |
As expected, the function works flawlessly for the specified color options.
Enhanced User Experience
A remarkable feature of ValidateSet is its ability to provide users with visual prompts. When you use a function with ValidateSet in an integrated scripting environment (ISE), you’ll notice that it generates a visual list of available options as you type. This dynamic feature significantly improves user interaction and minimizes input errors.
Moreover, when you’re working in the PowerShell console specify the -color parameter and then press the tab key, it auto-completes the available options. This not only saves time but also reduces the chances of input mistakes.
Limitations and Workarounds
While ValidateSet is a robust tool, it does have certain limitations that you should be aware of:
- Default Values: If you set a default value for a parameter outside the array of valid options, PowerShell won’t flag it as an error. For example, if you set a default color to ‘Black’ in our previous function, PowerShell won’t raise an error even though ‘Black’ is not in the ValidateSet. It’s essential to be cautious when setting defaults;
- Custom Error Messages: Another limitation is the inability to generate custom error messages within the function based on user input. However, you can address this by wrapping the function call in a Try/Catch block outside the function. This way, you can capture errors and execute specific error-handling code. Here’s an example:
function Write-Color { [cmdletbinding()] param( [Parameter()] [ValidateSet(‘Green’, ‘Blue’, ‘Red’)] [string] $color, $message ) Write-Host $message -ForegroundColor $color} Try { Write-Color -color Yellow -message “This will not work!”}Catch [System.Management.Automation.ParameterBindingException] { $errorMessage = $_.Exception.Message Write-Host “Error: [$errorMessage]” -ForegroundColor Red -BackgroundColor DarkBlue # Add your custom error-handling code here} |
In this example, the custom error message captures the issue when an invalid color is provided.
Video Guide
To finally answer all your questions, we have prepared a special video for you. Enjoy watching it!
Taking Parameter Validation to the Next Level
In the previous sections, we explored the power of the ValidateSet attribute in PowerShell to constrain the input of a parameter to a predefined set of values. This simple yet effective technique can enhance your scripts in various scenarios. However, PowerShell offers more advanced parameter validation options to take your scripting skills to the next level.
Let’s delve into some additional parameter validation techniques that can help you build robust and user-friendly scripts.
- ValidatePattern: Using Regular Expressions
While ValidateSet restricts input to a predefined list, ValidatePattern allows you to use regular expressions for more flexible validation. Regular expressions are patterns used to match character combinations in strings. By incorporating regular expressions into your parameter validation, you can enforce complex input requirements.
Here’s a quick example of how to use ValidatePattern:
function Validate-Email { [cmdletbinding()] param( [Parameter(Mandatory)] [ValidatePattern(“^\w+([-+.’]\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*$”)] [string]$email ) Write-Host “Valid email address: $email”} |
In this example, we validate whether the provided input matches the pattern of a valid email address.
- ValidateRange: Enforcing Numeric Ranges
ValidateRange is particularly useful when dealing with numeric parameters. It allows you to specify a minimum and maximum value to constrain input within a specific range. This ensures that your script receives valid numeric input.
Here’s an example of using ValidateRange:
function Validate-Age { [cmdletbinding()] param( [Parameter(Mandatory)] [ValidateRange(18, 99)] [int]$age ) Write-Host “Valid age: $age”} |
In this function, the age parameter is validated to ensure it falls within the range of 18 to 99.
- ValidateLength: Controlling String Length
When working with string parameters, you might want to enforce a specific length constraint. ValidateLength allows you to define the minimum and maximum length for string input.
Here’s an example:
function Validate-Password { [cmdletbinding()] param( [Parameter(Mandatory)] [ValidateLength(8, 20)] [string]$password ) Write-Host “Valid password: $password”} |
This function ensures that the provided password string is between 8 and 20 characters long.
- ValidateScript: Custom Validation Logic
Sometimes, predefined validation attributes might not cover your specific validation needs. In such cases, you can use ValidateScript to provide custom validation logic using a script block.
Here’s an example:
function Validate-Domain { [cmdletbinding()] param( [Parameter(Mandatory)] [ValidateScript({ if ($_ -match “^\w+\.(com|org|net)$”) { $true } else { throw “Invalid domain format: $_” } })] [string]$domain ) Write-Host “Valid domain: $domain”} |
In this function, we use a custom script block to validate whether the input matches a specific domain format.
Conclusion
PowerShell’s ValidateSet is a versatile and user-friendly feature that enhances the quality and usability of your scripts. By restricting parameter input to predefined values, you can minimize errors, improve script clarity, and create a more pleasant experience for users. While it has a few limitations, creative use of Try/Catch blocks can help you handle errors effectively. So, go ahead and incorporate ValidateSet into your PowerShell scripts to take full advantage of this powerful parameter validation tool.
Unleash the potential of ValidateSet in your PowerShell scripts, and watch your automation tasks become more robust and user-friendly. Happy scripting!