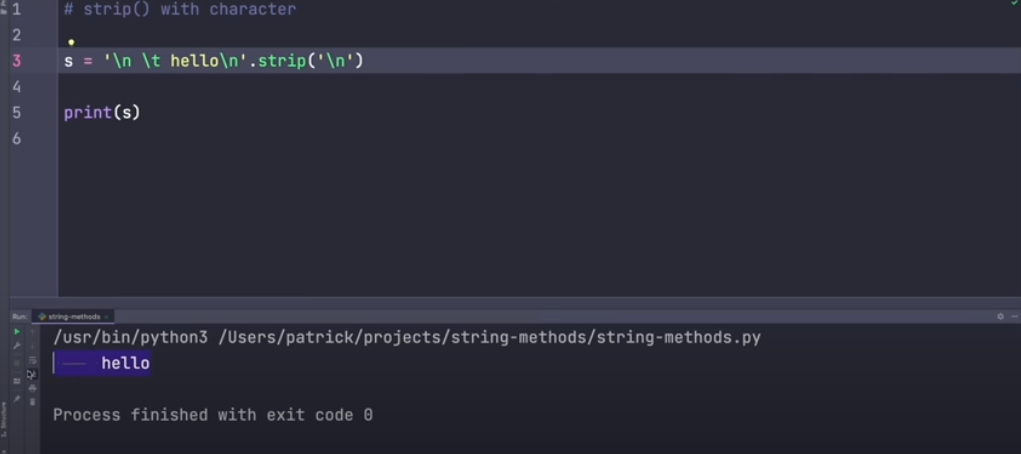
Python REST API Integration and Data Formatting
A journey starts with curiosity and a desire to learn something new. In this case, the adventure began with the protagonist’s girlfriend embarking on an exploratory trip.
Little did they know that this journey would lead them into the world of web scraping, data transformation, and Python scripting.
The Encounter
The research vessel where the protagonist’s girlfriend worked provided publicly accessible data that piqued their interest. The dataset included intriguing information such as temperature, depth, wind speed, latitude, and longitude, all accessible via the URL: webcam.oregonstate.edu/tracker. Initially, the hero thought about parsing web pages, but their attention was drawn to a convenient JavaScript feature on the site:
This JavaScript function optimized data retrieval. Of particular interest was the URL it used: app.uhds.oregonstate.edu/api/webcam/ship. Drawing from previous experience with PowerShell, the hero decided to conduct preliminary testing with it before transitioning to Python.
Initial Data Grab via PowerShell
The initial approach was simple yet effective. In PowerShell, the protagonist fetched data using the following code:
$url = 'https://app.uhds.oregonstate.edu/api/webcam/ship' $data = Invoke-RestMethod -Uri $url $data.count $data[0]
The result matched their expectations entirely.
Transitioning to Python
To tackle the same task in Python, several essential steps were necessary:
Data Retrieval
To obtain data, the hero utilized the requests library in Python.
import requests def get_data(): ship_api_url = "https://app.uhds.oregonstate.edu/api/webcam/ship" request_data = requests.get(ship_api_url) return request_data.json()
This function effectively retrieved the data and converted it into a Python dictionary.
Value Conversion
The hero needed to convert values from Celsius to Fahrenheit and from knots to mph. For this purpose, two functions were created:
def convert_c_to_f(temp): "Converts Celsius to Fahrenheit." conversion = round(9.0 / 5.0 * temp + 32, 2) return conversion def convert_knot_to_mph(knot): "Converts wind speed from knots to mph" conversion = round(1.1507 * knot, 2) return conversion
These functions ensured precision by rounding values to two decimal places.
String Formatting
In the final step, data needed to be formatted for presentation. A formatting function was developed:
def format_data(data_to_format): "Formats the data as desired" formatted = """ Air Temp [{0} F] Water Temp [{1} F] Wind [{2} mph] Depth [{3} meters] Lat [{4}] Long [{5}] Current Location: https://www.google.com/maps/place/{4},{5} *note* You may need to zoom out on the map to see the relative location! """.format(convert_c_to_f(data_to_format['air_temp']), convert_c_to_f(data_to_format['water_temp']), convert_knot_to_mph(data_to_format['wind']), data_to_format['depth'], data_to_format['lat'], data_to_format['lng']) return formatted
This function took data, applied necessary conversions, and structured it for display.
The End Result
Here’s the complete Python script:
import requests def get_data(): "Gets api data" ship_api_url = "https://app.uhds.oregonstate.edu/api/webcam/ship" request_data = requests.get(ship_api_url) return request_data.json() def convert_c_to_f(temp): "Converts Celsius to Fahrenheit." conversion = round(9.0 / 5.0 * temp + 32, 2) return conversion def convert_knot_to_mph(knot): "Converts wind from knots to mph" conversion = round(1.1507 * knot, 2) return conversion def format_data(data_to_format): "Formats the data how we want it" formatted = """ Air Temp [{0} F] Water Temp [{1} F] Wind [{2} mph] Depth [{3} meters] Lat [{4}] Long [{5}] Current Location: https://www.google.com/maps/place/{4},{5} *note* You may need to zoom out on the map to see the relative location! """.format(convert_c_to_f(data_to_format['air_temp']), convert_c_to_f(data_to_format['water_temp']), convert_knot_to_mph(data_to_format['wind']), data_to_format['depth'], data_to_format['lat'], data_to_format['lng']) return formatted data = get_data() latest_dataset = data[0] print(format_data(latest_dataset))
Running this script allowed them to obtain the data in the desired format.
How to Read Strings in Python?
In Python, there are several ways to read strings depending on specific requirements. Here are some common methods for reading strings:
Using the input() Function
The input() function is used to read strings from the user via the command line. It reads user input as a string. Here’s an example:
user_input = input("Enter a string: ") print("You entered:", user_input)
When this code is executed, the user will be prompted to enter a string, and the entered string will be stored in the variable user_input.
Reading from a File
To read a string from a file, you can utilize Python’s file-handling capabilities. Here’s an example of reading a string from a file:
# Open a file in read mode with open(‘example.txt’, ‘r’) as file: file_contents = file.read() print(“Contents of the file:”) print(file_contents)
In this example, the open() function is used to open a file in read mode (‘r’). Then, the read() method is used to read the contents of the file into a string.
Reading Multiple Lines from a File
If you need to read multiple lines of text from a file into a string, you can use a loop to iterate through the lines and concatenate them. Here’s an example:
# Open a file in read mode with open(‘example.txt’, ‘r’) as file: file_contents = file.read() print(“Contents of the file:”) print(file_contents)
This code reads each line from the file and appends it to the string file_contents.
Reading from Standard Input
You can also read strings from standard input (e.g., the command line) using the sys module. Here’s an example:
import sys user_input = sys.stdin.readline() print(“You entered:”, user_input)
These are the primary methods for reading strings in Python, and you can choose the one that best suits your needs. Each method has its advantages and is suitable for different scenarios.
Conclusion
The protagonist’s journey had two main objectives:
- Learning Python: They deepened their understanding of the Python language and its libraries by solving real-world problems;
- Bot Integration: The acquired knowledge was put to use in enhancing a Discord bot in Python. It now displays ship data along with the current webcam image, bridging the gap between data and visual effects.
In conclusion, this adventure showcased Python’s capabilities in working with REST APIs and data formatting, making it a valuable tool for both aspiring programmers and data enthusiasts.