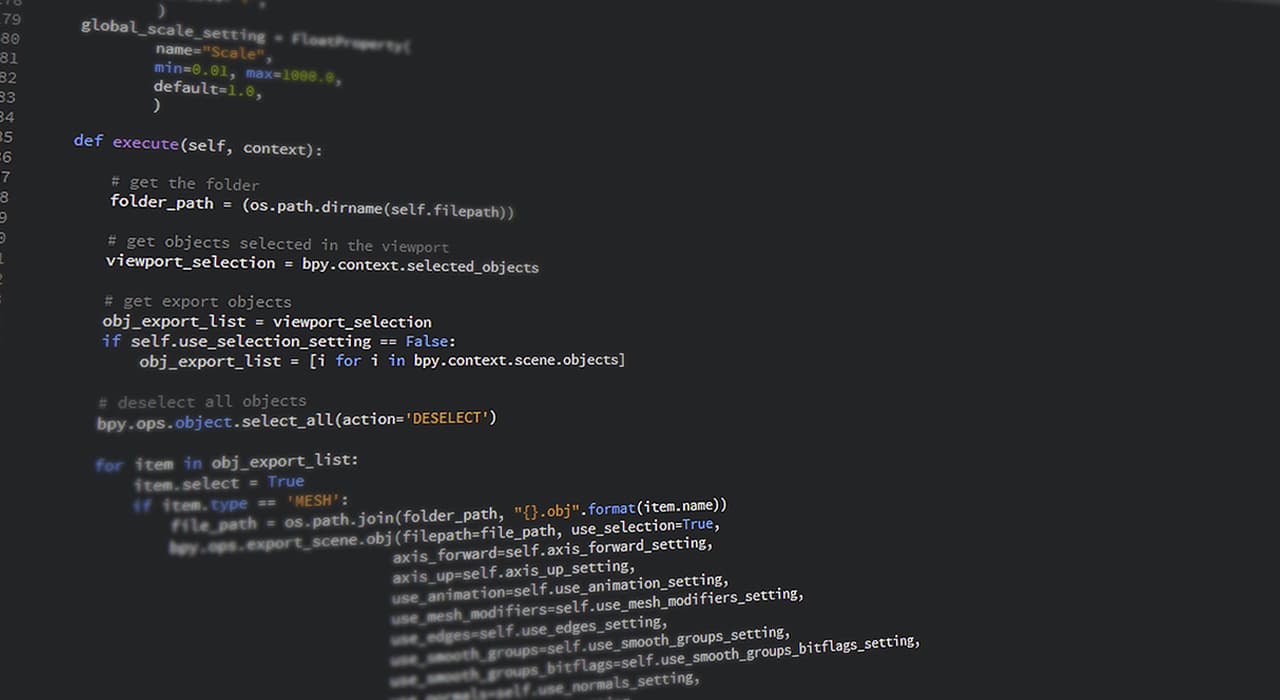
Realistic CLI example in Python using click
Now that you know how click makes writing the CLI easier, let’s look at a more realistic example. We’re going to write a program that allows us to interact with a webAPI.
The API we’re going to use next is the OpenWeatherMap API. It provides information about the current weather as well as a five-day forecast for a certain location. We’ll start with a test API that returns the current weather for a location.
Before we start writing code, let’s get familiar with the API. We can use the HTTPie service, including an online terminal.
Let’s see what happens when we access the API with London as the location:
$ http --body GET http://samples.openweathermap.org/data/2.5/weather \
q==London \
appid==b1b15e88fa797225412429c1c50c122a1
{
"base": "stations",
"clouds": {
"all": 90
},
"cod": 200,
"coord": {
"lat": 51.51,
"lon": -0.13
},
"dt": 1485789600,
"id": 2643743,
"main": {
"humidity": 81,
"pressure": 1012,
"temp": 280.32,
"temp_max": 281.15,
"temp_min": 279.15
},
"name": "London",
"sys": {
"country": "GB",
"id": 5091,
"message": 0.0103,
"sunrise": 1485762037,
"sunset": 1485794875,
"type": 1
},
"visibility": 10000,
"weather": [
{
"description": "light intensity drizzle",
"icon": "09d",
"id": 300,
"main": "Drizzle"
}
],
"wind": {
"deg": 80,
"speed": 4.1
}
}
If you’re confused by the API key in the example above, don’t worry, it’s a test API key provided by the service.
The more important observation is that we send two parameters (denoted by == when using HTTPie) to find out the current weather:
q – the location where we want to know the weather;
appid – our API key.
This allows us to create a simple Python implementation using the requests library (let’s omit error and failed requests handling for simplicity):
import requests
SAMPLE_API_KEY = 'b1b15e88fa797225412429c1c50c122a1'
def current_weather(location, api_key=SAMPLE_API_KEY):
url = 'http://samples.openweathermap.org/data/2.5/weather'
query_params = {
'q': location,
'appid': api_key,
}
response = requests.get(url, params=query_params)
return response.json()['weather'][0]['description']
This function makes a simple request to the API using two parameters. It takes location as a mandatory argument, which must be a string. We can also specify the API key by passing the api_key parameter when the function is called. This is optional, since the default is a test key.