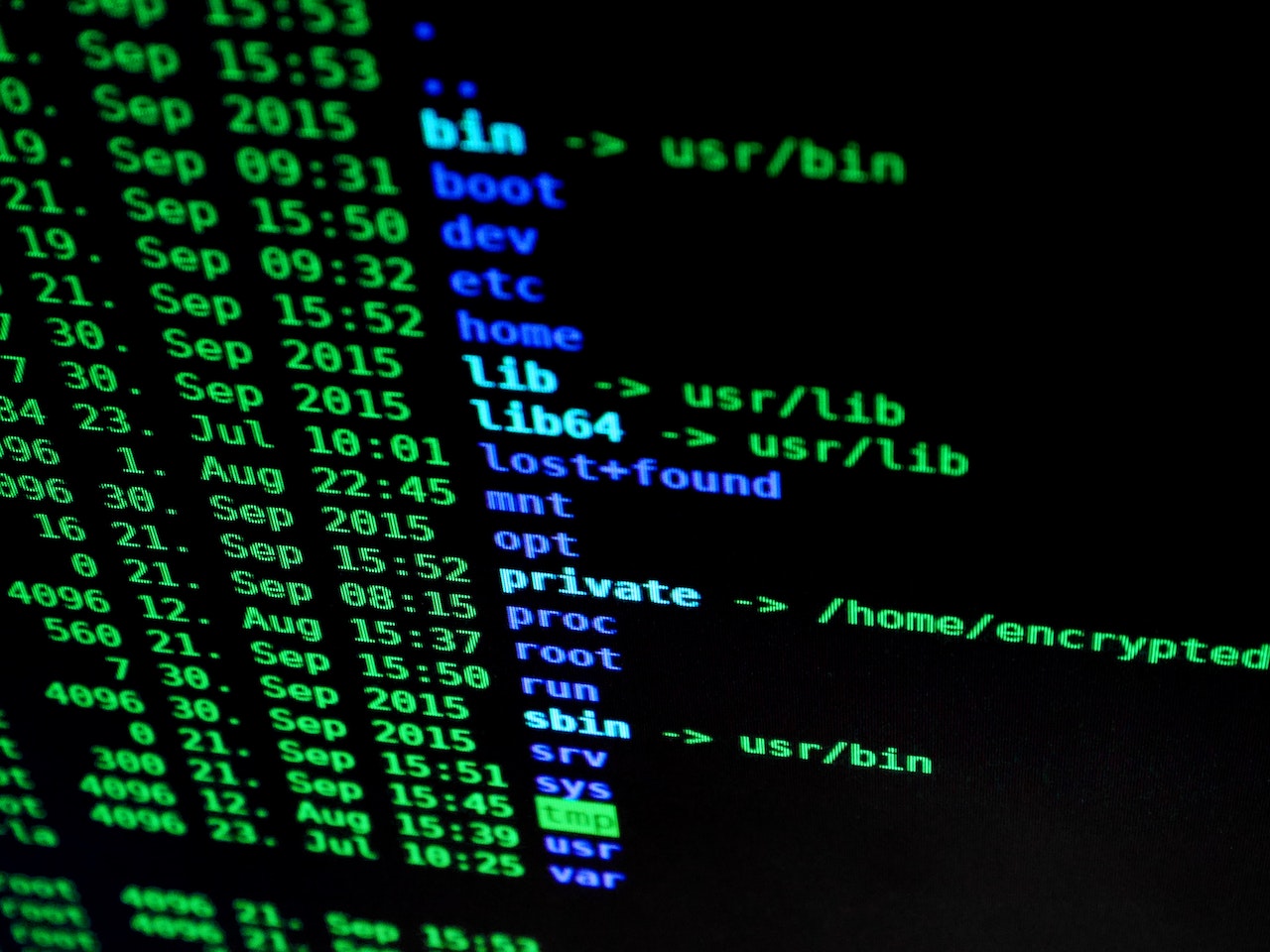
Building a Command Line Application With Node.js
Are you looking to take your coding skills to the next level? Developing a node command line application using Node.js CLI may be the challenge you need! A command line app enables users to interact with their computer or server by entering text-based commands, and learning how to build one from scratch is an invaluable skill.
This blog post will provide a detailed walkthrough of creating a practical command line application in Node.js CLI, including step-by-step instructions for laying out your project structure and helpful tips on debugging and optimizing performance. So get ready: it’s time to dive into building fully functioning command line apps with Node.js CLI!
What is a command-line interface (CLI)?
A command-line interface (CLI) is a text-based user interface for issuing commands to a computer. CLIs are often used by system administrators, programmers, and advanced users to give orders to the operating system, network services, and software programs.
A node CLI framework generally consists of a prompt (a message that indicates the user’s current location in the filesystem or program) and a command line (a text box where the user enters one or more commands). The user enters orders by typing them into the node command line and pressing Enter. The computer then executes the command.
Most CLIs include features that allow users to browse directories, view files, search for files, and create and delete files and directories. Some CLIs have advanced functions such as running programs, extracting files, and managing processes.
Prerequisites
Before getting started, make sure you have the following:
–Node.js CLI and npm installed on your computer
-A text editor such as Atom or Sublime Text
-Knowledge of JavaScript and Node js development fundamentals
-Understand basic command line operations (cd, ls, etc.)
-Familiarity with the Git version control system
Setting up a project structure
Once the prerequisites are in place, it’s time to start setting up the project structure for your command line application. Start by creating a directory named “my-cli”. Inside this directory, create two files – package.json and cli.js – and a folder called “commands.”
The package.json file stores information about your application, such as the name and version number. The cli.js file will contain the actual code for your command line app and will be our main entry point into the application. The commands folder will store all the individual commands users can issue using your CLI.
Within the “commands” folder, create a new directory called “helpers,” which will contain all of the helper functions used within your command line apps, such as input validation, argument parsing, and output formatting.
Now we’re ready to start writing code!
The Process of Creating a CLI tool
Creating a command line tool is reallbreaking down the task into small, manageable pieces. We’ll start by implementing the basic functionality that will allow users to execute commands in our CLI.
- Initialize and parse arguments
The first step is to initialize and parse any arguments that d in from the user. This can be done using Node’s built-in process object, which allows us to access command-line arguments within our application.
- Execute the command
Once the necessary arguments have been parsed, we can use these values to determine which command should be executed. This is usually done by matching a user-supplied value with an existing set of available commands stored in an array.
- Validate input
Once the appropriate command has been identified, we need to perform some basic validation on the inputs that were passed in. This can include checking for errors, verifying data types, and ensuring that required fields are present.
- Execute command logic
After validating the user’s input, we can execute the logic for our command by calling a helper function or running a predefined set of instructions.
- Format output
Finally, once the task has been completed and all necessary data has been generated, it needs to be formatted into a usable form so it can be displayed to the user. We can do this by using Node’s built-in console.log() method, which allows us to output data in various formats.
Once our essential command line tool is up and running, we can add more advanced features, such as support for multiple commands, custom colors and formatting, auto-completion, and more.
- Set up List Command
In addition to the basic setup we just covered, we can add a list command. This will allow us to easily view all available controls in our node CLI framework and display them in a nicely formatted table.
- Create a helper function
We’ll start by creating a helper function that queries our commands and returns an array of valid command names.
- Format output
After our helper function has been created, we can format our output into a nicely formatted table. This can be done by using Node’s built-in console.table() method allows us to quickly create and display tabular data in the terminal.
- Add list command
After our formatting is complete, we can add a new “list” command to our node CLI framework, allowing users to quickly view all available orders they can issue within our app.
Once these steps are completed, your primary command line application should be fully functional.
Test your CLI tool
Before releasing your CLI tool to the public, it’s essential to ensure everything works as expected. This can be done by testing your application with various inputs and scenarios.
1. Run unit tests
Unit tests are a great way to ensure that each app component functions appropriately in isolation. We can leverage Node’s built-in assert library to create basic unit tests for our command line tool, allowing us to quickly detect any bugs or issues before they become an issue for users.
2. Test commands manually
In addition to running automated tests, we should test our command line tool manually by issuing various commands and ensuring that the output is as expected. This will allow us to quickly identify any discrepancies or issues that automated tests may miss.
3. Mark-done command
Finally, we can add a “mark-done” command to our CLI, which allows us to quicklyasks as completed from within the terminal quickly by adding a new “done” command alongside our other bases will then update the task accordingly.
Publish the CLI tool
Once our CLI tool has been thoroughly tested, we can publish it to a package manager like NPM or Yarn. This will allow users to quickly and easily install and use our app in their projects.
1. Create a package file
Before publishing our app, we need to create a “package” file containing all of our application’s metadata, such as its name, version number, description, dependencies, etc.
2. Publish to NPM/Yarn
Once our package file is ready, we can publish our application to either NPM or Yarn by running the appropriate commands from the terminal.
3. Submit a pull request
Finally, once the package has been published, we should submit a pull request to the appropriate repository (e.g., NPM, Yarn) so our application can be accepted and officially released.
Don’t forget to update a CLI tool package regularly
Once our CLI tool has been released, it’s essential to regularly update the box with bug fixes and new features. This can be done by creating and submitting pull requests to the appropriate repository whenever necessary.
It’s also essential to keep our CLI tool up-to-date with the latest version of Node.js, as this will ensure that users can use our application without any issues. We can do this by keeping an eye on the Node.js release schedule and updating our package accordingly when new versions become available.
Conclusion
So how to use the command line? I hope you have seen it’s not that difficult to create and use command line tools. This node js command line tutorial covered creating a primary command line interface (CLI) using Node.js, including setting up commands, formatting output, testing functionality, and publishing the app on a package manager like NPM or Yarn. We also discussed why it’s essential to regularly update your CLI tool package to keep it up-to-date with the latest version of Node.js. Following these steps will help ensure that your CLI tool functions as expected and provides a great user experience for anyone using it.