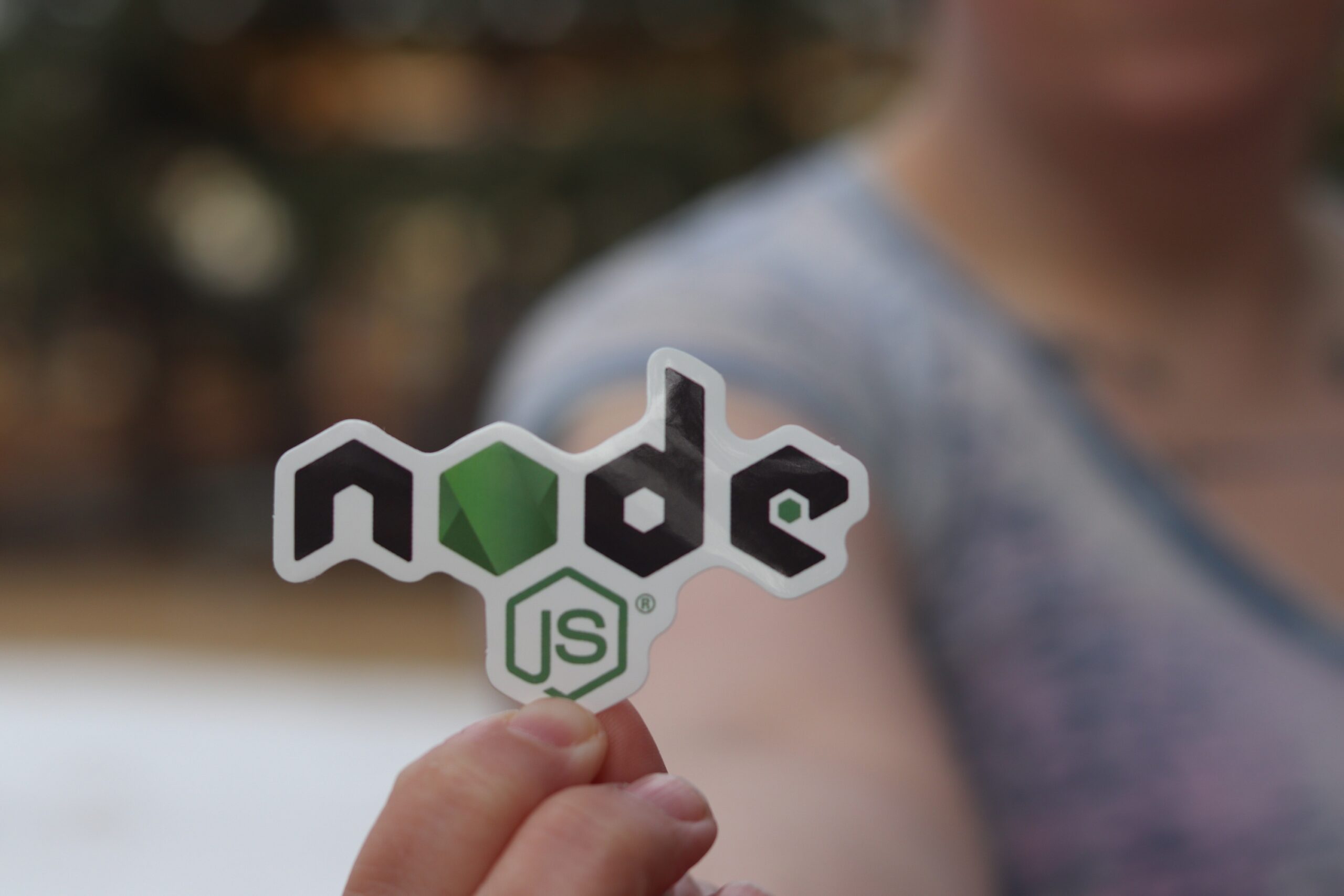
Mastering Command Line Development with Node.js
As a powerful and versatile runtime environment, Node.js has become increasingly popular among developers for its ability to streamline development and create scalable applications. While many developers use Node.js for web development, it can also be used for command line development to create powerful and efficient tools.
In this article, we will discuss the basics of Node.js for command line development, including how to set up your environment, create a new project, and start developing command line tools using Node.js. We will also provide some tips and tricks to make the development process more efficient.
Getting Started with Node.js for Command Line Development
Step 1: Install Node.js
The first step in getting started with Node.js for command line development is to install Node.js on your computer. You can download the latest version of Node.js from the official website and follow the installation instructions provided there.
Step 2: Set Up Your Environment
Once you have installed Node.js, the next step is to set up your environment. This includes configuring your command line interface (CLI) and setting up any necessary plugins or extensions. You may also want to install additional tools and libraries, such as npm (Node Package Manager), which can help you manage dependencies and streamline development.
Step 3: Create a New Project
With your environment set up, you can now create a new project. To create a new Node.js project for command line development, you can use the command-line tool “npm init”. This tool will guide you through the process of creating a new Node.js project, including setting up the project’s dependencies and configuring the project’s package.json file.
Step 4: Develop Your Command Line Tool
With your project created, you can now start developing your command line tool. This involves writing code, creating command line interfaces, and testing your tool on different operating systems and platforms. Make sure to follow best practices and guidelines for command line development to ensure that your tool is high-quality and meets the needs of your target audience.
Step 5: Deploy Your Command Line Tool
Once your tool is ready, the final step is to deploy it to your users. Depending on your specific requirements, this may involve distributing the tool through a package manager, such as npm, or providing users with a standalone executable. Make sure to follow the guidelines provided by the distribution channel and test your tool on different platforms before deploying it.
How to Automate Command Line Tasks with Node.js
If you’re a developer or system administrator, you know how time-consuming and tedious some command line tasks can be. Luckily, Node.js provides a way to automate these tasks, making your life easier and your workflow more efficient. In this article, we’ll discuss how to automate command line tasks with Node.js and provide some tips and tricks to help you get started.
Benefits of Automating Command Line Tasks with Node.js
Automating command line tasks with Node.js provides several benefits, including:
Saving time: Automating tasks can save you hours of manual work, especially for repetitive or complex tasks.
Reducing errors: By automating tasks, you can reduce the likelihood of human error, ensuring that your tasks are performed consistently and accurately.
Increasing efficiency: Automating tasks can help you streamline your workflow, allowing you to focus on more important tasks and projects.
Getting Started with Automating Command Line Tasks with Node.js
To get started with automating command line tasks with Node.js, you’ll need to install Node.js and the child_process module, which provides the ability to execute shell commands from within Node.js. Here’s how to install them:
- Install Node.js: Download and install the latest version of Node.js from the official website;
- Install the child_process module: Open your command line interface and enter the following command: npm install child_process.
Once you have Node.js and the child_process module installed, you can start automating your command line tasks.
Automating Command Line Tasks with Node.js
To automate a command line task with Node.js, you’ll need to create a Node.js script that executes the task. Here’s an example of how to automate the task of compressing a file using the gzip command:
const { exec } = require(‘child_process’); exec(‘gzip file.txt’, (error, stdout, stderr) => { if (error) { console.error(`exec error: ${error}`); return; } console.log(`stdout: ${stdout}`); console.error(`stderr: ${stderr}`); });
In this example, we use the exec function from the child_process module to execute the gzip command with the file.txt file. The error, stdout, and stderr parameters are callbacks that handle any errors, output, or error messages that occur during the execution of the command.
Tips and Tricks for Automating Command Line Tasks with Node.js
Here are some tips and tricks to help you automate command line tasks more efficiently:
Use command line arguments: To make your script more versatile, use command line arguments to specify file names, directory paths, and other variables that can be passed to the script at runtime.
Use control flow modules: Control flow modules, such as async or Promise, can help you manage complex workflows by allowing you to execute tasks in parallel, sequence, or in other patterns.
Use error handling: Make sure to handle errors properly to ensure that your script doesn’t fail unexpectedly. Use try-catch blocks or error-handling functions to catch and handle errors that may occur during the execution of your script.
When it comes to designing and developing command line interfaces (CLI), there are several best practices that can help ensure that your CLI is user-friendly, efficient, and easy to use. Whether you are developing a CLI for a custom application, a utility tool, or any other purpose, following these best practices can help you create a high-quality user experience.
One of the most important aspects of CLI design is simplicity. Your CLI should have a clear and concise structure that makes it easy for users to navigate and use. This means using simple and intuitive commands and options, avoiding unnecessary complexity, and providing helpful documentation and examples.
Another important aspect of CLI design is consistency. Your CLI should use consistent terminology, syntax, and formatting throughout, so that users can easily understand and remember how to use it. This includes using consistent command and option names, using clear and concise descriptions, and formatting output in a consistent and easily readable way.
Usability is also a key consideration in CLI design. Your CLI should be designed with the user’s needs and preferences in mind, and should be easy and efficient to use. This means providing helpful error messages, providing tab completion for commands and options, and using clear and concise prompts and messages.
Finally, testing and debugging are crucial components of CLI development. Your CLI should be thoroughly tested and debugged to ensure that it is working as intended and that there are no errors or bugs that could impact the user experience. This includes testing the CLI on different operating systems, ensuring that it is compatible with different versions of dependencies, and making sure that it is secure and resistant to common attack vectors.
By following these best practices, you can create a CLI that is efficient, user-friendly, and easy to use. Whether you are developing a CLI for a custom application or a utility tool, taking the time to design and develop it with best practices in mind can help ensure its success and improve the user experience.
Best Practices for Command Line Interface Design and Development
When it comes to designing and developing command line interfaces (CLI), there are several best practices that can help ensure that your CLI is user-friendly, efficient, and easy to use. Whether you are developing a CLI for a custom application, a utility tool, or any other purpose, following these best practices can help you create a high-quality user experience.
One of the most important aspects of CLI design is simplicity. Your CLI should have a clear and concise structure that makes it easy for users to navigate and use. This means using simple and intuitive commands and options, avoiding unnecessary complexity, and providing helpful documentation and examples.
Another important aspect of CLI design is consistency. Your CLI should use consistent terminology, syntax, and formatting throughout, so that users can easily understand and remember how to use it. This includes using consistent command and option names, using clear and concise descriptions, and formatting output in a consistent and easily readable way.
Usability is also a key consideration in CLI design. Your CLI should be designed with the user’s needs and preferences in mind, and should be easy and efficient to use. This means providing helpful error messages, providing tab completion for commands and options, and using clear and concise prompts and messages.
Finally, testing and debugging are crucial components of CLI development. Your CLI should be thoroughly tested and debugged to ensure that it is working as intended and that there are no errors or bugs that could impact the user experience. This includes testing the CLI on different operating systems, ensuring that it is compatible with different versions of dependencies, and making sure that it is secure and resistant to common attack vectors.
By following these best practices, you can create a CLI that is efficient, user-friendly, and easy to use. Whether you are developing a CLI for a custom application or a utility tool, taking the time to design and develop it with best practices in mind can help ensure its success and improve the user experience.
Conclusion
Node.js is a powerful and versatile runtime environment that can be used for both web development and command line development. By following the step-by-step guide and tips and tricks provided in this article, you can get started with Node.js for command line development and create high-quality and efficient command line tools. If you are looking for professional help with custom Node js development, we at Orangesoft can provide you with the expertise and experience you need to create custom applications that meet your specific business needs. Contact us today to learn more.