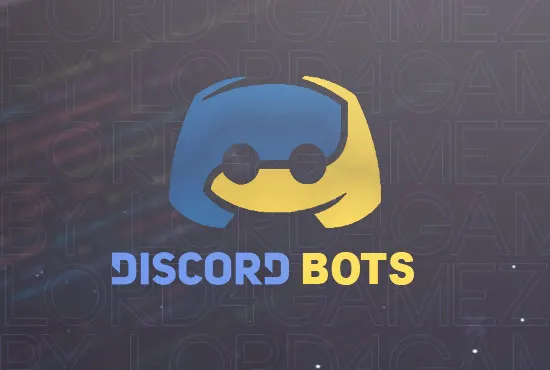
C# Discord Bot: Command Handling
Are you ready to level up your C# Discord bot game? Command handling is a crucial aspect of building robust and user-friendly bots that can perform a variety of tasks in Discord servers. In this comprehensive guide, we will delve deep into the world of C# Discord bot command handling, exploring everything from the basics to advanced techniques. By the end of this article, you’ll be equipped with the knowledge and skills to create a powerful and responsive Discord bot that stands out in any server.
Understanding the Basics of Command Handling
What Are Discord Bot Commands?
Discord bot commands are special messages that trigger specific actions or responses from your bot. They are essentially the way users interact with your bot, making them a fundamental component of any Discord bot.
Setting Up Your Development Environment
Before we dive into the coding part, let’s make sure you have everything set up for a seamless development experience. Here are the essential tools you’ll need:
- IDE (Integrated Development Environment): You can use Visual Studio or Visual Studio Code for C# bot development;
- Discord Developer Portal: Create a bot application on the Discord Developer Portal and obtain your bot token;
- C# Discord Library: We’ll be using the Discord.Net library for building our bot.
Creating Your First Command
Now, let’s get our hands dirty by creating our very first bot command. We’ll create a simple “Hello” command that makes the bot respond when a user types “!hello” in the server.
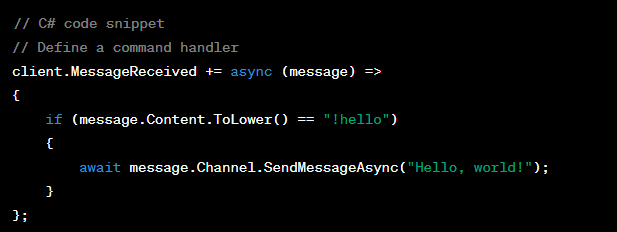
Advanced Command Handling Techniques
Command Parameters
In more complex scenarios, you may need to create commands that accept parameters. For example, a command that searches for information or performs actions based on user input.
Here’s how you can handle command parameters:
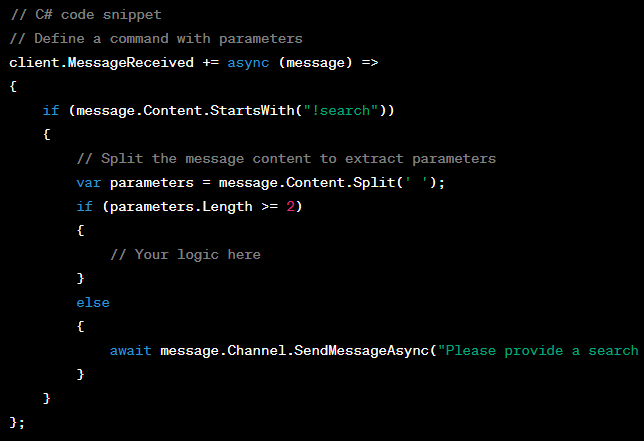
Organizing Your Commands
Command Modules and Categories
As your bot grows, it’s essential to keep your commands organized. Command modules and categories help you structure your bot’s functionality. For instance, you can have separate modules for moderation, fun, utility, and more.
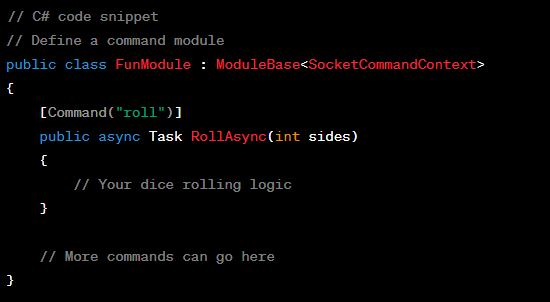
Handling Errors Gracefully
In real-world scenarios, errors are bound to happen. Whether it’s a user mistake or an unexpected issue, handling errors gracefully is crucial for providing a smooth user experience.
Exception Handling
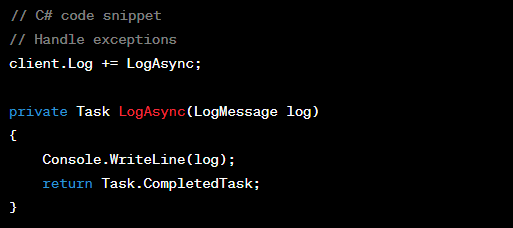
Enhancing User Experience
Creating a bot that responds promptly and effectively to user commands is essential for a positive user experience. To achieve this, consider the following techniques:
- Response Formatting: Ensure that your bot’s responses are well-formatted and easy to read. Use line breaks and proper indentation for multi-line responses;
- Response Time: Aim for minimal response times. Long delays can frustrate users. Optimize your code to handle commands swiftly, even in high-traffic servers;
- Interactive Commands: Implement interactive commands that allow users to provide input or make choices within the conversation. This can make interactions more engaging and dynamic.
Implementing Permissions and Security
When developing a Discord bot, it’s crucial to maintain server security and respect user privacy. Here’s how you can achieve this:
- Permission Checks: Implement permission checks to ensure that only authorized users can execute certain commands. Discord provides a range of permission levels, from server owner to regular members;
- Sensitive Data Handling: If your bot interacts with sensitive information, such as user data or authentication tokens, ensure that this data is handled securely. Use encryption and secure storage practices;
- Rate Limiting: To prevent abuse and spam, consider implementing rate limiting for certain commands. This restricts the number of times a command can be used within a specific time frame.
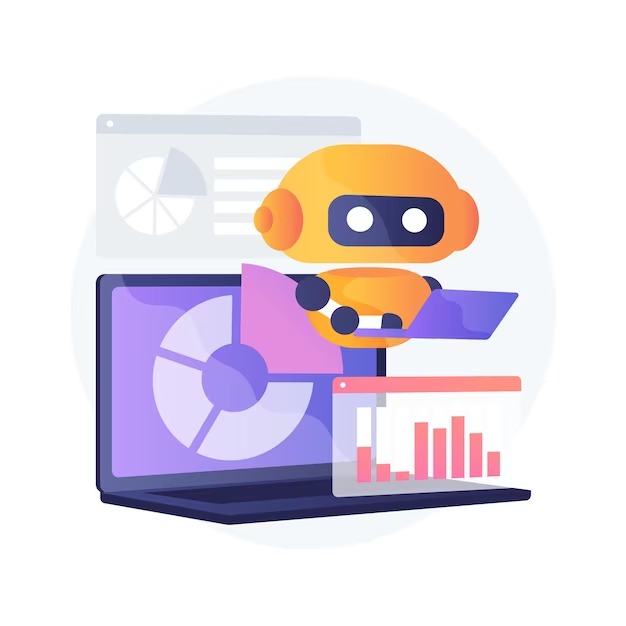
Scaling Your Bot
As your bot becomes more popular and serves multiple servers, you’ll need to scale it effectively. Here are some strategies for scaling your C# Discord bot:
- Sharding: Discord bot sharding involves splitting your bot’s functionality across multiple instances (shards). This helps distribute the load and ensures your bot can handle a large number of servers and users;
- Database Optimization: If your bot relies on a database to store data or user preferences, optimize your database queries and consider using caching mechanisms to reduce load times;
- Monitoring and Analytics: Implement monitoring tools to keep an eye on your bot’s performance and user engagement. Analytics can provide insights into which commands are most popular and help you make data-driven decisions.
Keeping Your Bot Updated
Discord is a dynamic platform, and maintaining your bot is an ongoing process. Here are some tips for keeping your C# Discord bot up to date:
- Library Updates: Regularly update your Discord library (e.g., Discord.Net) to stay compatible with the latest Discord API changes and benefit from bug fixes and improvements;
- Bot Features: Listen to user feedback and consider adding new features or refining existing ones based on user needs and trends in the Discord community;
- Bug Fixes: Address and fix any reported bugs promptly. Users appreciate a responsive and actively maintained bot;
- Community Engagement: Engage with your bot’s user community through platforms like Discord servers or forums. This can help you gather feedback and build a loyal user base.
Customizing Command Responses
Embed Messages
To enhance the visual appeal of your bot’s responses, consider using Discord’s embed messages. These messages allow you to format information in a structured and aesthetically pleasing way. You can include titles, descriptions, fields, and even images within an embed message.
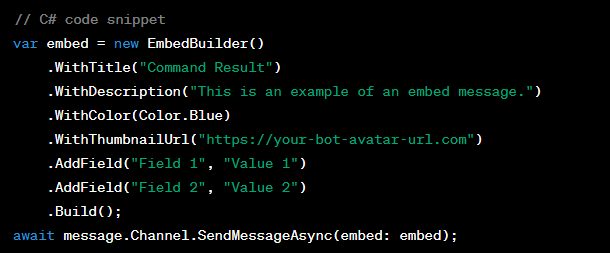
Rich Text Formatting
Discord supports a variety of text formatting options, allowing you to make your bot’s responses more engaging. You can use Markdown-style formatting to create bold text, italicize content, or even create clickable links. Here are some examples:
- Bold Text: **This text is bold**;
- Italic Text: *This text is italicized*;
- Clickable Link
Implementing Advanced Command Logic
Command Cooldowns
In situations where you want to prevent users from spamming a specific command, you can implement command cooldowns. Cooldowns specify a time period during which a user cannot use the same command again.
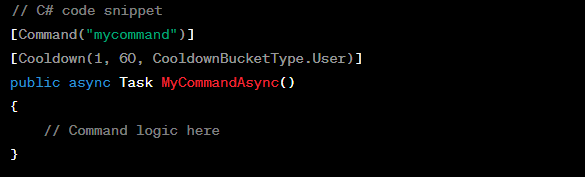
Command Permissions
For more granular control over who can use specific commands, you can assign permissions to commands. This ensures that only users with the required roles or permissions can access certain bot functionalities.
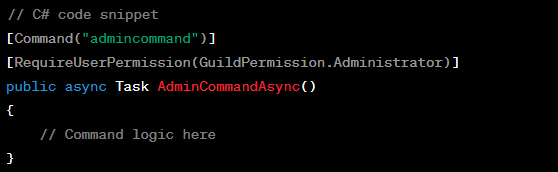
Handling Command Errors Effectively
User-Friendly Error Messages
When a user encounters an error while using your bot, it’s essential to provide clear and user-friendly error messages. These messages should guide users on what went wrong and how to rectify it.
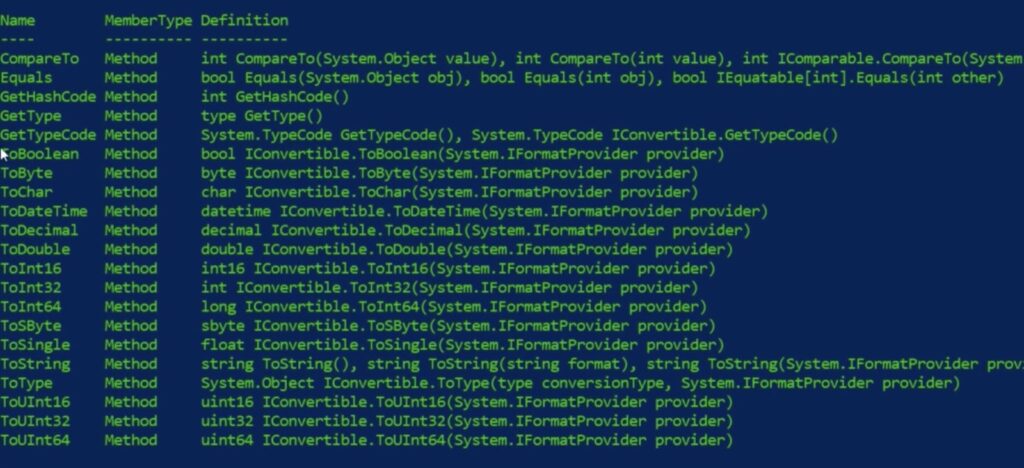
Logging Errors
In addition to informing users of errors, it’s crucial to log errors on your end for debugging and troubleshooting purposes. Implement a robust logging system to capture and record errors, including details like timestamps and user IDs.
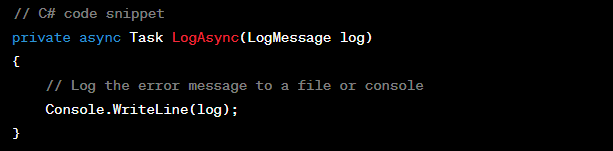
Conclusion
Mastering C# Discord bot command handling is a significant milestone in your journey as a bot developer. We’ve covered the basics, advanced techniques, organization strategies, and error handling to equip you with a solid foundation. Now, it’s your turn to experiment, create, and enhance your Discord bot’s capabilities. With dedication and creativity, you can build a bot that becomes an indispensable part of any Discord community.
FAQs
A Discord bot command is a special message that users can send to a bot to trigger specific actions or responses. It’s a way for users to interact with the bot and request various tasks or information.
To develop a C# Discord bot, you’ll need an IDE like Visual Studio or Visual Studio Code, access to the Discord Developer Portal to create a bot application, and the Discord.Net library for bot development.
You can handle command parameters by parsing the content of the user’s message. Split the message content to extract parameters and then process them accordingly within your command handler.
Error handling is crucial in Discord bot development to ensure a smooth user experience. It helps prevent crashes and provides informative responses to users when something goes wrong.
You can explore online communities, forums, and Discord bot development documentation for more resources and support in your C# Discord bot development journey.