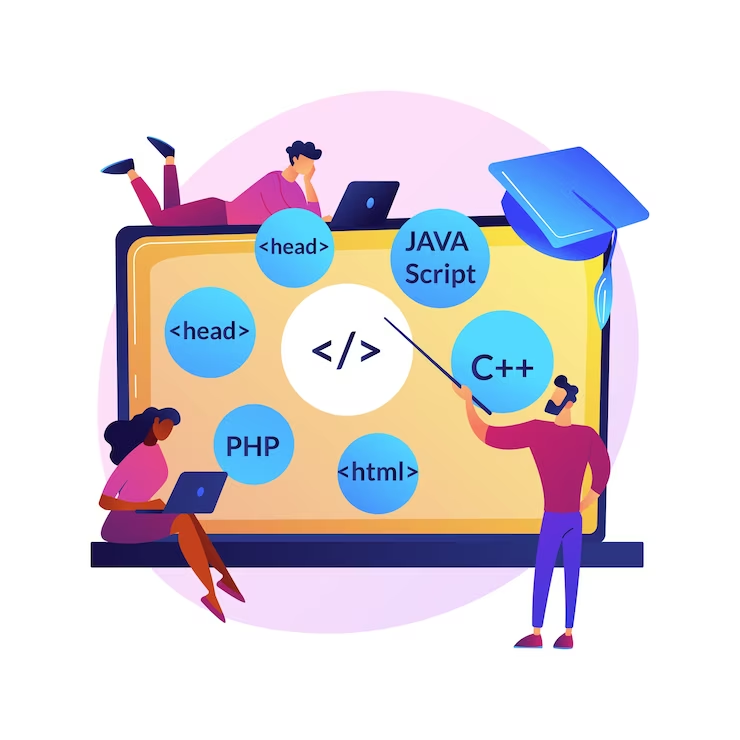
PowerShell Class: Hierarchies for Enhanced Scripting
Since PowerShell 5, you can harness the capabilities of custom classes in your PowerShell scripts.
While classes are a common feature in many programming languages, they are not always necessary in PowerShell. In most cases, custom objects suffice for your scripting needs.
Creating Your Custom Class
To define a class in PowerShell, you use the `class` keyword. Below is an example of a simple class definition, along with instances of that class created using both the `new-object` cmdlet and the `new()` keyword.
```powershell
class Tree {
[int]$Height
[int]$Age
[string]$Color
}
$tree1 = new-object Tree
$tree2 = [Tree]::new()
$tree1.Height = 10
$tree1.Age = 5
$tree1.Color = "Red"
$tree2.Height = 20
$tree2.Age = 10
$tree2.Color = "Green"
$tree1
$tree2
```
The result is:
```
Height Age Color
------ --- -----
10 5 Red
20 10 Green
```
Constructors: Initializing Your Class
Constructors are special methods called when you create an instance of a class. They are useful for initializing properties. You can define multiple constructors for a class, allowing you to customize object creation.
```powershell
class Tree {
[int]$Height
[int]$Age
[string]$Color
Tree() {
$this.Height = 1
$this.Age = 0
$this.Color = "Green"
}
Tree([int]$Height, [int]$Age, [string]$Color) {
$this.Height = $Height;
$this.Age = $Age;
$this.Color = $Color;
}
}
$tree1 = [Tree]::New()
$tree2 = New-Object Tree 5, 2, "Red"
$tree1
$tree2
```
The result is:
```
Height Age Color
------ --- -----
1 0 Green
5 2 Red
```
Methods: Adding Functionality
While you can manipulate class properties directly, methods offer a way to encapsulate actions. In this example, we create a `Grow` method to simulate a tree’s growth.
```powershell
class Tree {
[int]$Height
[int]$Age
[string]$Color
Tree() {
$this.Height = 1
$this.Age = 0
$this.Color = "Green"
}
Tree([int]$Height, [int]$Age, [string]$Color) {
$this.Height = $Height
$this.Age = $Age
$this.Color = $Color
}
[void]Grow() {
$heightIncrease = Get-Random -Min 1 -Max 5
$this.Height += $heightIncrease
$this.Age += 1
}
}
$tree = [Tree]::New()
# Let the tree grow for 10 years
for ($i = 0; $i -lt 10; $i++) {
$tree.Grow()
$tree
}
```
The result shows the tree’s growth over 10 years:
```
Height Age Color
------ --- -----
3 1 Green
7 2 Green
10 3 Green
11 4 Green
15 5 Green
16 6 Green
20 7 Green
24 8 Green
26 9 Green
28 10 Green
```
Discover more about Class, Objects in this video
Class Inheritance: Building on Foundations
While classes can be used similarly to custom objects, they shine when it comes to inheritance.
The result demonstrates class inheritance:
```powershell
class Tree {
[int]$Height
[int]$Age
[string]$Color
Tree() {
$this.Height = 1
$this.Age = 0
$this.Color = "Green"
}
Tree([int]$Height, [int]$Age, [string]$Color) {
$this.Height = $Height
$this.Age = $Age
$this.Color = $Color
}
[void]Grow() {
$heightIncrease = Get-Random -Min 1 -Max 5
$this.Height += $heightIncrease
$this.Age += 1
}
}
class AppleTree : Tree {
[string]$Species = "Apple"
}
$tree = [AppleTree]::new()
$tree
```
```
Species Height Age Color
------- ------ --- -----
Apple 1 0 Green
```
Conclusion
PowerShell classes offer flexibility and customization for your scripts. Whether you’re creating custom objects or harnessing the power of inheritance, understanding classes can enhance your scripting capabilities.