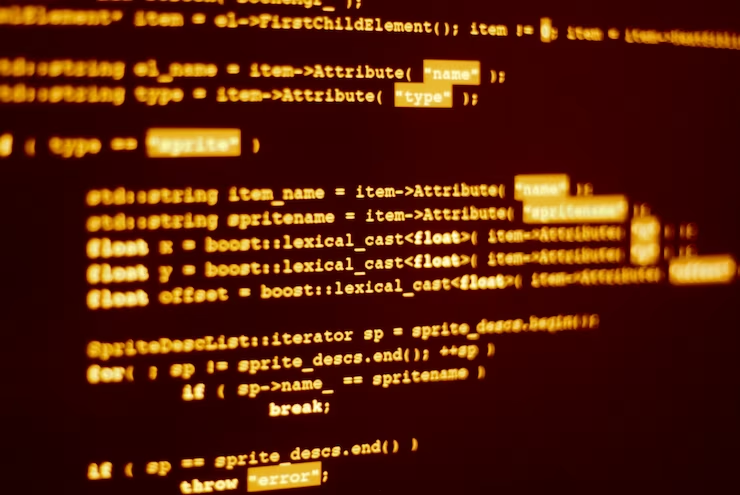
PowerShell Sort Array: Tips and Tricks for Mastering
Sorting arrays is a fundamental skill for any PowerShell scripter. Whether you’re organizing data, analyzing logs, or streamlining file handling, knowing how to sort arrays efficiently can significantly enhance your scripting capabilities. In this guide, we’ll dive deep into PowerShell array sorting, covering various sorting techniques and sharing valuable tips along the way.
PowerShell Array Sorting Basics
Before we delve into specific sorting techniques, let’s get acquainted with the basics of array sorting in PowerShell. PowerShell provides the `Sort-Object` cmdlet, your go-to tool for sorting arrays. By default, `Sort-Object` performs an ascending, case-insensitive sort. Here’s a quick overview of the default behavior:
```powershell
$names = @("Muffin", "Romeo", "Noodle", "Zoe", "Jack", "Luna", "Gracie", "mittens", "Phoebe", "Peanut", "Harley", "Jake")
$names | Sort-Object
```
The result:
```
Gracie
Harley
Jack
Jake
Luna
mittens
Muffin
Noodle
Peanut
Phoebe
Romeo
Zoe
```
Now that we’ve covered the basics let’s explore more advanced sorting scenarios.
Sorting in Descending Order
By default, `Sort-Object` arranges data in ascending order. To sort in descending order, simply use the `-Descending` parameter:
```powershell
$names | Sort-Object -Descending
```
The result:
```
Zoe
Romeo
Phoebe
Peanut
Noodle
Muffin
mittens
Luna
Jake
Jack
Harley
Gracie
```
Sorting with Case Sensitivity
PowerShell’s default sorting is case-insensitive. However, if you need case-sensitive sorting, the `-CaseSensitive` parameter comes to the rescue:
```powershell
$names = @("Muffin", "muffin", "Noodle", "Zoe", "zoe", "Luna", "Gracie", "peanut", "Phoebe", "Peanut", "Harley", "Jake")
$names | Sort-Object -CaseSensitive
```
The result:
```
Gracie
Harley
Jake
Luna
muffin
Muffin
Noodle
peanut
Peanut
Phoebe
zoe
Zoe
```
Sorting Unique Values
Sorting arrays often involves eliminating duplicates. PowerShell makes this a breeze with the `-Unique` parameter:
```powershell
$names = @("Muffin", "muffin", "Noodle", "Zoe", "zoe", "Luna", "Gracie", "peanut", "Phoebe", "Peanut", "Harley", "Jake")
$names | Sort-Object -Unique
```
The result:
```
Gracie
Harley
Jake
Luna
muffin
Noodle
peanut
Peanut
Phoebe
zoe
Zoe
```
Check more tutorials about Array and Arraylist here
Sorting by Property
In PowerShell, objects often come with properties. You can sort an array of objects based on these properties using the `-Property` parameter:
```powershell
$files = Get-ChildItem -Path $env:TEMP
$files | Sort-Object -Property LastWriteTime | Where-Object { $_.PSIsContainer -eq $false }
```
This command sorts files in the `$env:TEMP` directory based on the `LastWriteTime` property, filtering out directories (containers).
Additional Tips for Efficient Sorting
- Custom Sorting: You can implement custom sorting logic using script blocks with `Sort-Object`. This allows you to sort arrays based on your specific requirements;
- Optimize Performance: When working with large datasets, specifying an initial capacity for arrays can enhance performance. PowerShell’s default hashtable capacity is 8, but you can adjust it to suit your needs.
Conclusion
Sorting arrays in PowerShell is a crucial skill for scripters and administrators. Whether you’re working with lists of names, file directories, or complex datasets, PowerShell’s `Sort-Object` cmdlet empowers you to arrange and manage data efficiently. By mastering these array sorting techniques, you’ll streamline your scripting tasks and become more proficient in harnessing the power of PowerShell.