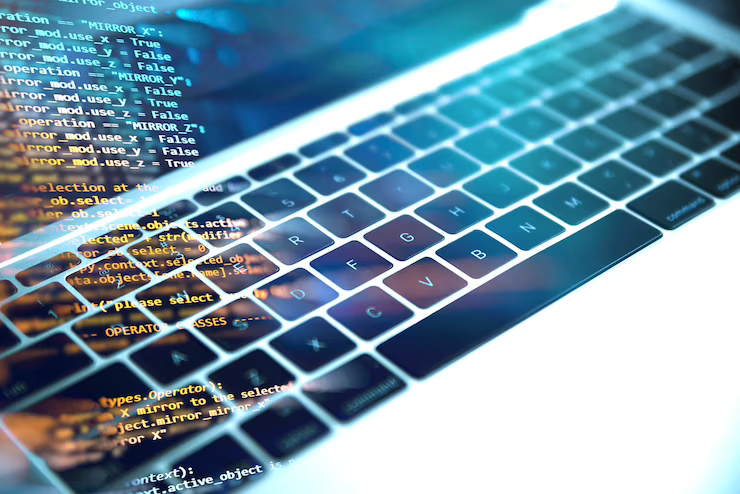
PowerShell Hashtable: Techniques for Efficient Scripting
PowerShell, a powerful scripting language, equips you with a plethora of data structures to efficiently manage and manipulate data. Among these, Hashtables stand out as versatile and indispensable tools for any PowerShell scripter.
In this comprehensive guide, we will explore PowerShell Hashtables, delving into their purpose, structure, practical applications, and advanced techniques.
What is Hashtable in PowerShell?
In PowerShell, a Hashtable is a fundamental data structure used for storing and retrieving data in a key-value pair format. This means that for each unique key, there is an associated value. Hashtables are particularly efficient for data retrieval as they utilize a hashing algorithm to swiftly locate values based on their corresponding keys.
The key (no pun intended) advantage of using Hashtables lies in their ability to provide quick access to data without the need to iterate through all elements, as you would with an array or list. This makes Hashtables an invaluable tool for managing data efficiently, especially in scenarios where you need to access specific information rapidly.
How to Create a Hashtable in PowerShell?
Creating a Hashtable in PowerShell is a straightforward process. You enclose the key-value pairs within curly braces `{}` and separate them using colons `:`.
Here’s the basic syntax:
$myHashtable = @{
"Key1" = "Value1"
"Key2" = "Value2"
"Key3" = "Value3"
}
Let’s break down the steps:
- Begin with the variable assignment symbol `$`;
- Assign a variable name to your Hashtable, such as `$myHashtable`;
- Use the assignment operator `=`;
- Open a pair of curly braces `{}` to define the Hashtable;
- Inside the braces, specify the key and its associated value, separated by a colon `:`. You can add as many key-value pairs as needed, each on a new line;
- Close the curly braces `{}`.
Here’s a practical example:
$employee = @{
"Name" = "John"
"Age" = 35
"Department" = "IT"
}
In this example, we’ve created a Hashtable named `$employee` with three key-value pairs: “Name” maps to “John,” “Age” maps to 35, and “Department” maps to “IT.”
Once your Hashtable is created, you can access its values using the associated keys. For instance, to retrieve the employee’s name, you would use:
$employee["Name"] # This will return "John"
This simple syntax and efficient data retrieval capability make Hashtables a powerful asset in PowerShell scripting and data management.
For more tips watch this tutorial Powershell Hashtables
Now, let’s delve deeper into advanced techniques and best practices for working with Hashtables in PowerShell.
Advanced Techniques and Best Practices for Hashtables in PowerShell
Hashtables are incredibly versatile and offer various advanced techniques for optimizing your PowerShell scripts. Here are some best practices and advanced techniques:
Adding and Updating Entries
To add a new key-value pair to an existing Hashtable, simply assign a value to a new or existing key.
```powershell
$myHashtable["NewKey"] = "NewValue"
```
To update an existing entry, assign a new value to an existing key.
```powershell
$myHashtable["ExistingKey"] = "UpdatedValue"
```
Checking if a Key Exists
You can check if a key exists in a Hashtable using the `ContainsKey()` method.
```powershell
if ($myHashtable.ContainsKey("Key1")) {
Write-Host "Key1 exists!"
}
```
This conditional check helps you avoid errors when attempting to access a key that might not exist in the Hashtable.
Removing Entries
To remove a key-value pair from a Hashtable, use the `Remove()` method.
```powershell
$myHashtable.Remove("Key2")
```
This allows you to clean up your Hashtable when a key is no longer needed.
Cloning Hashtables
When assigning one Hashtable to another variable, PowerShell creates a reference to the original Hashtable, meaning that changes to one will affect the other. If you need a true copy of a Hashtable, you can use the `Clone()` method.
```powershell
$original = @{
"Name" = "John"
"Age" = 30
}
$copy = $original.Clone()
$copy["Age"] = 31
```
In this example, the `$original` Hashtable remains unchanged, while `$copy` is updated independently. This is useful when you want to preserve the original data.
Enumerating Hashtables
You can iterate through the keys and values of a Hashtable using a `foreach` loop. This allows you to perform actions on each key-value pair.
```powershell
$myHashtable = @{
"Name" = "John"
"Age" = 30
"City" = "New York"
}
foreach ($key in $myHashtable.Keys) {
$value = $myHashtable[$key]
Write-Host "$key: $value"
}
```
This code snippet iterates through the keys of `$myHashtable`, retrieves the corresponding values, and displays them. It’s a handy way to process all elements in your Hashtable.
Hashtable Capacity
By default, a Hashtable in PowerShell has a capacity of 8, which means it can efficiently store up to 8 key-value pairs. If you anticipate needing to store more data, consider specifying an initial capacity when creating the Hashtable. This can significantly improve performance.
```powershell
$myHashtable = @{} # Default capacity of 8
$largeHashtable = New-Object 'System.Collections.Hashtable' 50 # Capacity of 50
```
This becomes particularly relevant when dealing with larger datasets, as it helps prevent the need for Hashtable resizing, which can impact performance.
What Are Hashtables?
At its core, a Hashtable is a data structure that enables you to store and retrieve data using key-value pairs. It’s akin to a dictionary in Python or an associative array in other programming languages. In PowerShell, Hashtables are enclosed in curly braces `{}` and consist of key-value pairs separated by colons `:`.
```powershell
$myHashtable = @{
"Key1" = "Value1"
"Key2" = "Value2"
"Key3" = "Value3"
}
```
Hashtables are exceptionally efficient when it comes to data retrieval. Unlike arrays that require iterating through all elements to find a specific value, Hashtables employ a hashing algorithm to swiftly locate the value associated with a given key.
Practical Applications of Hashtables
Hashtables find utility in various scenarios, including:
- Configuration Settings. Hashtables serve as excellent containers for storing configuration settings for your scripts or applications. This simplifies retrieval and modification of settings without the need for complex logic.
```powershell
$settings = @{
"Server" = "example.com"
"Port" = 8080
"Timeout" = 30
}
```
- Counting Occurrences. Hashtables excel at counting occurrences of elements within a dataset. You can use keys to represent unique elements and values to store their counts.
```powershell
$fruits = @{
"Apple" = 5
"Banana" = 3
"Orange" = 7
}
```
- Grouping Data. Hashtables enable you to group data based on specific criteria. This proves particularly valuable when dealing with complex datasets.
```powershell
$employees = @(
@{ "Name" = "John"; "Department" = "HR" },
@{ "Name" = "Sarah"; "Department" = "Finance" },
@{ "Name" = "Mike"; "Department" = "IT" }
)
$groupedEmployees = @{}
$employees | ForEach-Object {
$department = $_.Department
if ($groupedEmployees.ContainsKey($department)) {
$groupedEmployees[$department] += @($_)
} else {
$groupedEmployees[$department] = @($_)
}
}
```
Tips for Working with PowerShell Hashtables
Adding and Updating Entries
To add a new key-value pair to a Hashtable, simply assign a value to a new or existing key.
```powershell
$myHashtable["NewKey"] = "NewValue"
```
To update an existing entry, assign a new value to an existing key.
```powershell
$myHashtable["ExistingKey"] = "UpdatedValue"
```
Checking if a Key Exists
You can check if a key exists in a Hashtable using the `ContainsKey()` method.
```powershell
if ($myHashtable.ContainsKey("Key1")) {
Write-Host "Key1 exists!"
}
```
Removing Entries
To remove a key-value pair from a Hashtable, use the `Remove()` method.
```powershell
$myHashtable.Remove("Key2")
```
Conclusion
PowerShell Hashtables are a versatile data structure that can significantly enhance your scripting and automation tasks. By understanding their fundamentals, practical applications, and advanced techniques, you can wield Hashtables with precision and efficiency.
Whether you’re managing configuration settings, counting occurrences, grouping data, or performing more complex data operations, Hashtables are a valuable asset in your PowerShell toolkit.
Hashtables offer efficient data storage and retrieval, making them an essential tool for scripters and administrators working with PowerShell. Mastering Hashtables will empower you to tackle a wide range of scripting challenges effectively, ultimately streamlining your automation efforts.