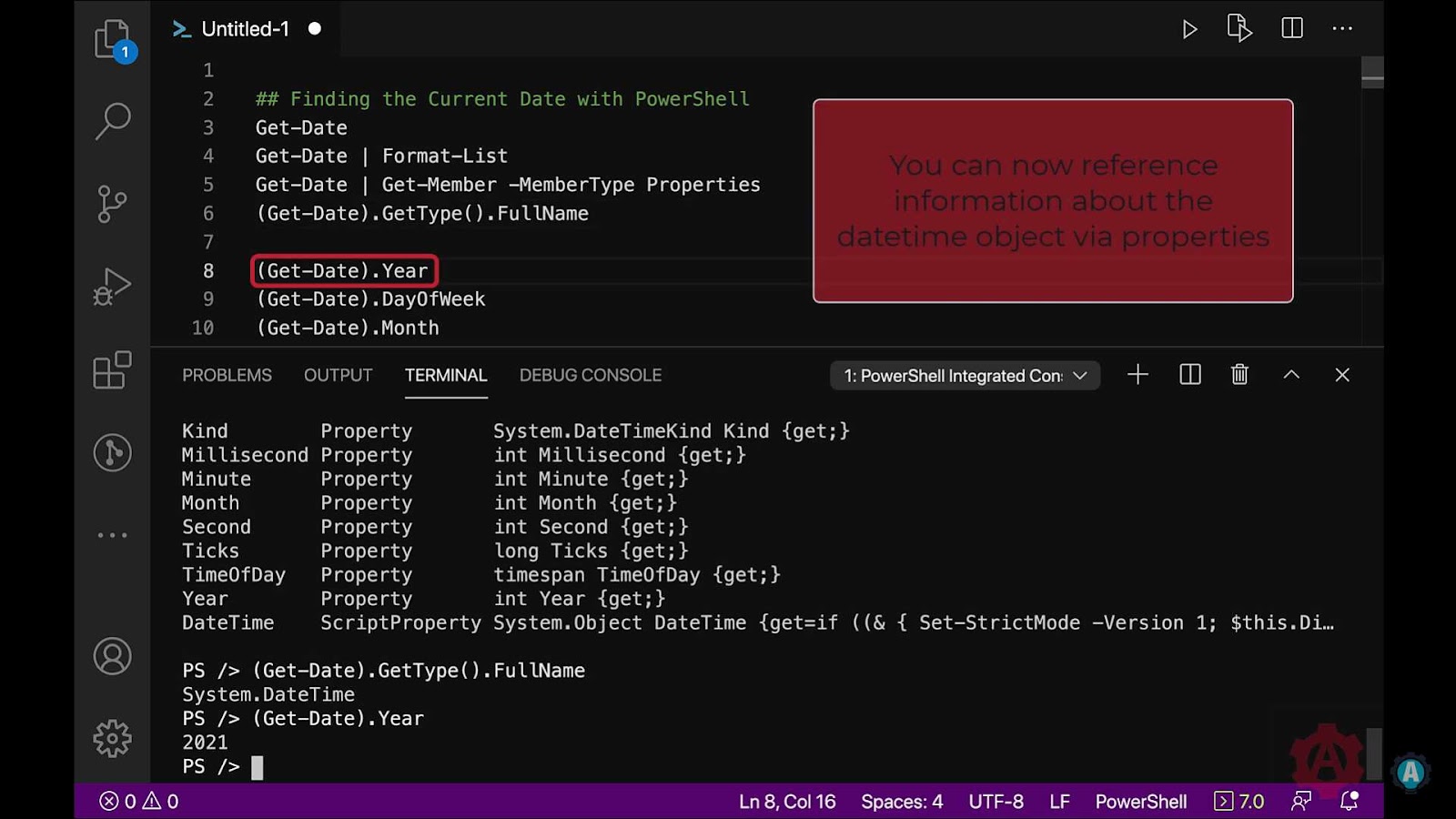
Exploring Date and Time Manipulation in PowerShell
In numerous PowerShell operations, there arises a recurrent necessity for acquiring time and date data. This entails the utilization of the [datetime] data type when retrieving date and time values. The specific format in which these values are delivered hinges on the cultural preferences configured within the computer’s settings.
Retrieving Current Date and Time
Acquiring the current date and time can be easily accomplished by utilizing the Get-Date command. This command is pivotal for those who need to manage and analyze time-related data efficiently.
Detailed Description
When the Get-Date command is employed, it fetches the present date and time, generating a result that may look like the following:
Wednesday, September 21, 2023 2:30:00 PM
This output can be extremely beneficial for various applications such as scripting, system monitoring, and data logging, facilitating accurate time stamping and scheduling tasks.
Extracting Specific Date and Time Components
For a more granular approach, one can extract specific components of the date and time, employing properties within the [datetime] type. This is essential when there is a need to isolate and analyze individual parts of the datetime object for meticulous examinations or specific adjustments.
Comprehensive Procedure
To extract individual components, assign the Get-Date command to a variable, for example, $today, and then utilize Write-Host to display specific properties. Here is a generalized illustration of the method:
$today = Get-Date
Write-Host "Comprehensive Date: `t$today"
Write-Host "Date Only: `t`t$($today.Date)"
Write-Host "Year: `t`t$($today.Year)"
Write-Host "Month: `t`t$($today.Month)"
Write-Host "Day: `t`t$($today.Day)"
Write-Host "Day of the Week: $($today.DayOfWeek)"
Write-Host "Day of the Year: $($today.DayOfYear)"
Write-Host "Hour: `t`t$($today.Hour)"
Write-Host "Minute: `t$($today.Minute)"
Write-Host "Second: `t$($today.Second)"
Write-Host "Millisecond: $($today.Millisecond)"
When executed, the script will display detailed information, for instance:
Comprehensive Date: 09/21/2023 14:30:00
Date Only: 09/21/2023 00:00:00
Year: 2023
Month: 9
Day: 21
Day of the Week: Wednesday
Day of the Year: 264
Hour: 14
Minute: 30
Second: 00
Millisecond: 500
Applications and Insights
Understanding and implementing these techniques is essential, particularly for developers and system administrators, as they enable refined manipulation and analysis of date and time data. For instance, by isolating specific datetime components, users can generate more readable and customized outputs, perform precise calculations and comparisons, or formulate intricate scheduling algorithms. These operations can be particularly advantageous in enhancing the functionality and efficiency of software applications, scripts, and system management tasks.
Formatting Dates for Clarity and Precision
In a world where information is omnipresent and easily accessible, the manner in which data is presented becomes crucial. Proper representation and formatting of dates ensure smooth comprehension and interpretation of data. The -f parameter plays a pivotal role in this process, allowing individuals to mold date displays according to their preferences or project requirements.
Detailed Overview
When working with dates, the necessity of formatting is often underscored. The significance of structured and well-formatted dates cannot be overemphasized as they help in conveying information in an unambiguous and coherent manner, eliminating the risks of misunderstandings and errors.
The -f parameter serves as a catalyst in the transformation of date representation. It accepts strings and modifies the placeholders, adjusting them to align with the values obtained from the datetime type. It enables a clear, concise, and systematic presentation of dates, facilitating easier readability and comprehension.
Example and Result
Consider a scenario where Get-Date is utilized to obtain the current date:
$date = Get-Date
When executed, $date typically reveals the complete date and time:
Monday, March 14, 2022, 4:47:03 PM
In this instance, applying the -f parameter with the desired format string, such as “yyyyMMdd”, allows the user to customize the date representation:
$date -f "yyyyMMdd"
Subsequently, the outcome would appear succinct, displaying only the necessary components, like so:
20220314
This manipulation enables users to extract and present data in a more tailored and effective manner, matching specific needs or preferences.
Expanded Utility and Implications
The use of the -f parameter in date formatting can be invaluable in a multitude of applications. From data analysis, management, and presentation to software development, the ability to customize date representations enables professionals to communicate and interpret temporal information efficiently. This customization is crucial in maintaining clarity and reducing the possibility of misinterpretations due to varying date format standards across different regions and industries.
The versatile -f parameter accommodates a broad spectrum of format strings, allowing users to explore different combinations and representations, tailoring the output to their distinct requirements. It thus aids in the creation of more dynamic and adaptable solutions, enhancing the precision and accessibility of date-related data.
Manipulating Dates with PowerShell: A Comprehensive Guide
1. Performing Arithmetic Operations on Dates
In PowerShell, manipulating dates through arithmetic operations, such as addition and subtraction, is attainable and versatile. Initially, these operations might seem a bit perplexing; however, they are reasonably straightforward once understood. For addition, one would use positive numbers, and for subtraction, negative numbers are employed.
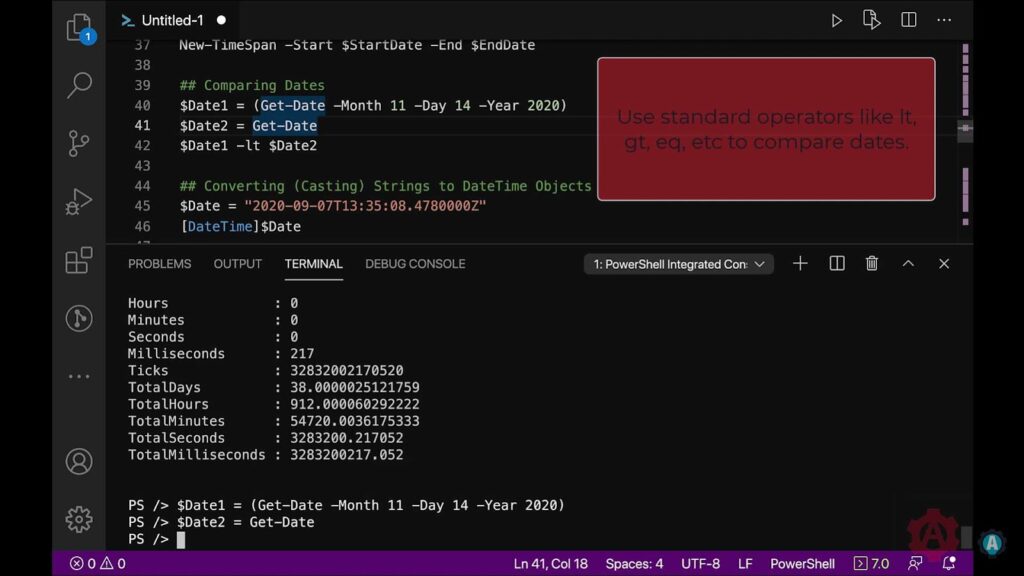
Consider the following code snippet as an example:
$today = Get-Date
$yesterday = $today.AddDays(-1)
$tomorrow = $today.AddDays(1)
Write-Host "Today: $today"
Write-Host "Yesterday: $yesterday"
Write-Host "Tomorrow: $tomorrow"
Here, $today is assigned the current date and time. To calculate the date for yesterday and tomorrow, the .AddDays() method is used with parameters -1 and 1 respectively, indicating subtraction and addition of one day to the current date. When executed, this code will yield the following:
Today: MM/DD/YYYY HH:MM:SS
Yesterday: MM/DD/YYYY HH:MM:SS
Tomorrow: MM/DD/YYYY HH:MM:SS
Beyond days, PowerShell also offers a plethora of functions allowing for the addition or subtraction of different time units. Here are some of the functions available:
Add (Method: datetime Add(timespan value))
AddDays (Method: datetime AddDays(double value))
AddHours (Method: datetime AddHours(double value))
AddMilliseconds (Method: datetime AddMilliseconds(double value))
AddMinutes (Method: datetime AddMinutes(double value))
AddMonths (Method: datetime AddMonths(int months))
AddSeconds (Method: datetime AddSeconds(double value))
AddTicks (Method: datetime AddTicks(long value))
AddYears (Method: datetime AddYears(int value))
These functions are paramount for developers and system administrators who need to manipulate time intervals and perform date arithmetic for various purposes like scheduling tasks and managing logs.
2. Assigning Specific Dates and Times
Assigning and manipulating specific dates and times in PowerShell is accomplished through the Set-Date command. This command permits the user to explicitly set the date and time to a desired value, offering high precision and customization in date manipulation. For instance:
$date = Get-Date -Date "YYYY-MM-DD HH:MM:SS"
$date
Executing this command assigns a specific date and time to the $date variable, and the result will be displayed in a format similar to: Day, Month DD, YYYY HH:MM:SS AM/PM.
Further, PowerShell allows for modification of individual components of the current date. For example, to change only the month of the current date, the following command is used:
$date = get-date -Month X
$date
In this example, replacing X with the desired numerical month will yield a new date with the modified month, while the day, year, and time remain unchanged. This feature is highly beneficial for those who need to adjust specific elements of dates, allowing for enhanced flexibility and precision in date and time management.
Conclusion
Mastering date manipulations in PowerShell can significantly augment productivity for
developers and system administrators. Whether performing arithmetic operations on dates, assigning specific dates and times, or modifying individual components of dates, PowerShell provides an array of functions and commands to satisfy diverse needs. It empowers users to handle dates and times with increased flexibility, precision, and efficiency, ultimately contributing to optimized task scheduling, log management, and many other aspects of system administration and development.