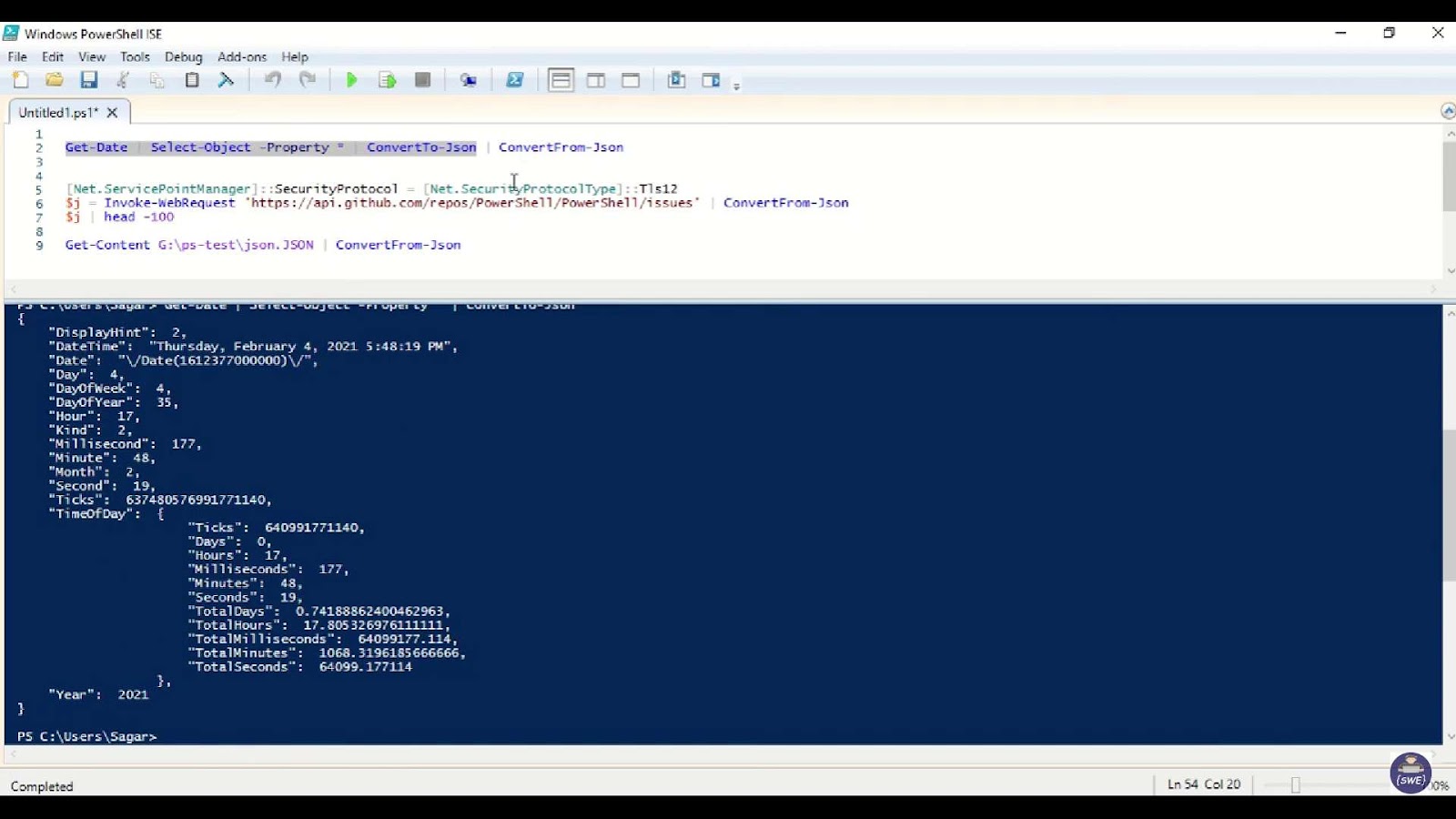
Unlocking the Power of JSON Transformation
When it comes to PowerShell, one of its standout capabilities is its proficiency in managing JSON data. PowerShell’s remarkable adaptability in dealing with custom objects empowers users to effortlessly and potently manipulate JSON data, rendering the task a seamless and potent endeavor.
Retrieving and Processing JSON Data
JSON (JavaScript Object Notation) is a lightweight data-interchange format that’s easy for humans to read and write and easy for machines to parse and generate. One might need to parse JSON data for a variety of reasons, such as obtaining configuration settings or displaying data in applications.
Utilizing the ConvertFrom-Json Command:
In the realm of PowerShell, when it comes to deciphering JSON data, the ConvertFrom-Json cmdlet emerges as an indispensable tool. This particular command wields the power to metamorphose a JSON string into a tailored PowerShell entity, thereby rendering the data considerably more amenable for subsequent manipulation and stylish presentation.
Fetching Data from a URL:
In some cases, the JSON data may be stored on a remote server and needs to be fetched first. To do so, PowerShell offers an approach via the System.Net.WebClient class.
For example:
$url = "https://sample-url.com/sample.json"
$jsonContent = (New-Object System.Net.WebClient).DownloadString($url)
Once the JSON content is fetched, it’s time to convert it:
$parsedData = $jsonContent | ConvertFrom-Json
Accessing Specific Data:
Having parsed the data, specific sections can be accessed. To obtain color details:
$parsedData.colors
This might yield an output similar to:
color category type code
----- -------- ---- ----
black hue primary @{rgba=System.Object[]; hex=#000}
white value @{rgba=System.Object[]; hex=#FFF}
red hue primary @{rgba=System.Object[]; hex=#FF0}
... and so forth
Comprehending the Output:
The data subjected to parsing undergoes a metamorphosis, taking on the form of bespoke PowerShell entities. Even data nested beyond a solitary tier is meticulously accommodated: when data delves deeper than one level, it undergoes a metamorphosis into a unique entity, nestling snugly within its progenitor. For instance, consider the illustration above, where the “code” attribute, housing both rgba and hex values, is transmuted into its distinct custom entity, ensconced within the overarching “color” entity.
This process guarantees that users can effortlessly access data nested at any depth. Nonetheless, to seamlessly navigate the labyrinth of nested entities, one must possess familiarity with the underlying data structure.
Encoding Data in JSON Format
When working with datasets like the one in the mentioned illustration, it’s often necessary to revert the processed data back to its standard JSON format, especially for tasks like storage or data exchange. To achieve this transformation, the ConvertTo-Json command is utilized.
Steps to Encode Data into JSON and Store in a File:
Conversion: Before diving into this process, ensure that the original dataset or the modified version of it is available for use. Once this is ascertained, harness the power of the ConvertTo-Json command to transform the data.
$data | ConvertTo-Json
Storing Data: After converting the data to its JSON format, the next step is to store this data in a file. PowerShell provides the Out-File command, which effectively writes the converted data to a desired location.
$data | ConvertTo-Json | Out-File $env:temp\json.txt -Force
Verification: It’s always a good practice to verify if the data was stored correctly. Use the Get-Content command to retrieve the content of the file:
Get-Content $env:temp\json.txt
On successful completion of the above steps, the output in the file should resemble:
{
"colors": [
{
"color": "black",
"category": "hue",
"type": "primary",
"code": "@{rgba=System.Object[]; hex=#000}"
},
{
"color": "white",
"category": "value",
"code": "@{rgba=System.Object[]; hex=#FFF}"
},
...
]
}
A Note on Data Integrity:
While the above method is effective in converting and storing data, it’s imperative to ensure the integrity of the stored data. In the aforementioned example, an inconsistency was observed: the color codes weren’t stored as plain strings. Instead, they retained their imported type. Such nuances can lead to data discrepancies and need attention. Always inspect the final data format and ensure it aligns with the expected structure, making necessary modifications if required.
Converting Data to JSON with Custom Depth
The depth of data when converting to JSON is an essential factor to consider, especially in tools such as PowerShell. This depth determines how many nested levels of the data structure will be represented in the outputted JSON format.
Understanding Default Depth:
In certain PowerShell versions, particularly PowerShell 5, the default depth for conversion is 2. However, this default setting might not be immediately evident to the user, as there isn’t a built-in mechanism to notify them that data might be truncated. Thus, users might occasionally overlook important nested data.
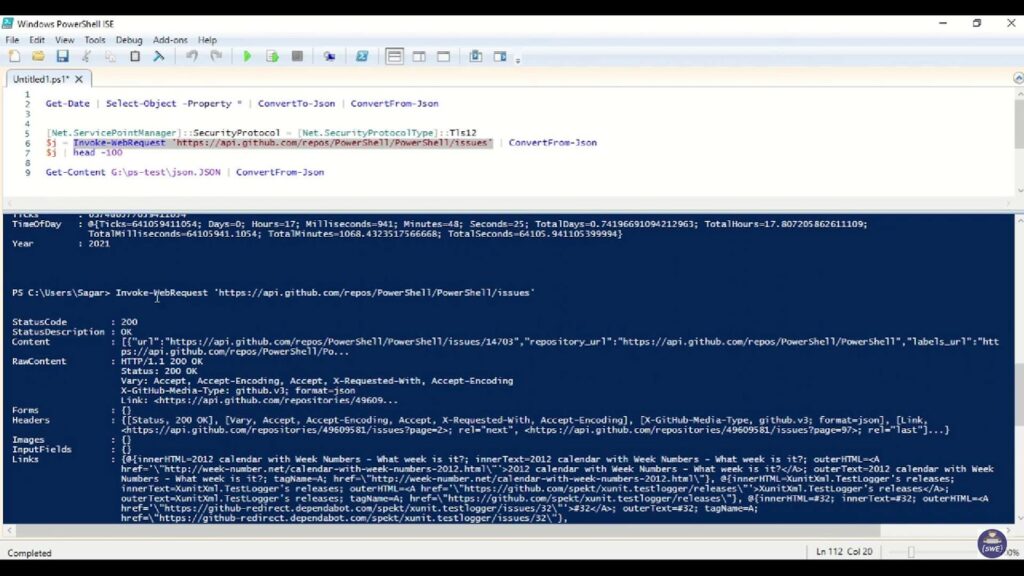
On the other hand, PowerShell 7 enhances user experience by providing an explicit warning if there is potential for data truncation due to the depth limit. This serves as a cue to remind the user that they might need to adjust the depth parameter to capture all the necessary information.
Adjusting the Depth Parameter:
To achieve a more tailored JSON conversion, PowerShell offers the -Depth parameter, which allows users to define the desired depth of their output. For instance, consider a dataset that requires a depth of 3 for an accurate representation. The following command could be employed:
$data | ConvertTo-Json -Depth 3 | Out-File $env:temp\json.txt -Force
Example Outcome:
When the data is converted using the appropriate depth, the output will be more comprehensive. Take the following JSON output as an illustration:
{
"colors": [
{
"color": "black",
"category": "hue",
"type": "primary",
"code": {
"rgba": "255 255 255 1",
"hex": "#000"
}
},
{
"color": "white",
"category": "value",
"code": {
"rgba": "0 0 0 1",
"hex": "#FFF"
}
},
...
]
}
The depth ensures that even the innermost nested data (like ‘rgba’ and ‘hex’ codes in the example above) are properly represented, resulting in a comprehensive and accurate JSON output.
Conclusion
When engaging in JSON transformations within the realm of PowerShell, grasping the intricacies of the depth parameter and skillfully configuring it can prove to be the pivotal factor distinguishing a mere partial data depiction from a comprehensive one. Vigilance in inspecting the depth parameter is of paramount importance, particularly when navigating intricate nested datasets, ensuring the attainment of an optimal outcome is secured.