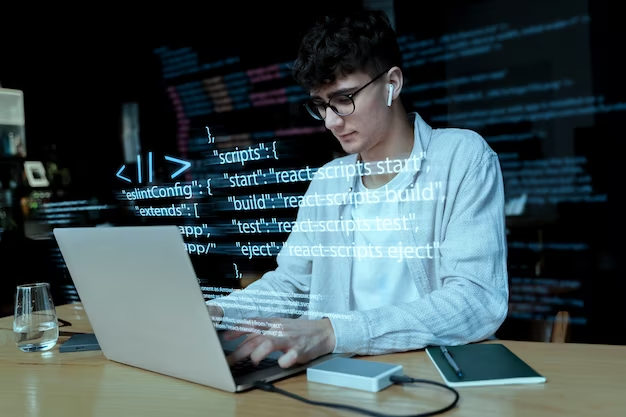
Mastering PowerShell Strings: Formatting, and Scriptblocks
In the world of PowerShell, everything is an object, including strings. In this comprehensive guide, we’ll delve into the fascinating realm of PowerShell strings. From basic operations to advanced manipulation techniques, you’ll gain a deep understanding of how to harness the power of strings in PowerShell.
What is a String in PowerShell?
As mentioned in Part 1, PowerShell treats everything as an object. Today, our focus is on one specific object type: System.String. In PowerShell, a string is essentially an object with the type System.String.
Let’s begin our exploration by examining how to work with strings in PowerShell.
Getting Started with Strings
We’ll start with a simple example. Consider the following command:
$ourPath = Get-Location |
Here, we use the Get-Location cmdlet to retrieve the current location, and we store it in the variable $ourPath.
To understand what’s stored in $ourPath, we can simply echo its value:
$ourPath |
Now, let’s dig deeper into the object type, methods, and properties associated with $ourPath by using the Get-Member cmdlet:
$ourPath | Get-Member |
You’ll discover that the object type of $ourPath is System.Management.Automation.PathInfo. To obtain the string value contained within this object, we need to access the Path property:
$ourPath.Path |
Now that we’ve identified the string value, let’s move on to string manipulation.
String Manipulation
String manipulation is a fundamental skill in PowerShell. We’ll demonstrate various techniques to manipulate strings effectively.
Substring Method
The SubString method allows you to extract a portion of a string based on its position. It takes two numerical arguments: the starting position and the length of the substring.
For example, to retrieve the first 8 characters of a string:
$ourPath.Path.SubString(0, 8) |
To get the string “part9” from the path “C:\PowerShell\part9”:
$ourPath.Path.SubString(14, 5) |
LastIndexOf Method
The LastIndexOf method helps locate the position of the last occurrence of a specified character within a string. This is particularly useful when dealing with file paths.
To find the position of the last backslash (\) in a path:
$ourPath.Path.LastIndexOf(‘\’) |
By combining the SubString and LastIndexOf methods, you can automatically extract the name of the child folder from a path:
$childFolderName = $ourPath.Path.SubString($ourPath.Path.LastIndexOf(‘\’) + 1) |
This technique simplifies the extraction of folder names from paths.
Expanded Strings
Expanded strings, enclosed in double quotes, allow you to interpolate variable values directly within a string. For example:
$test = ‘This is a test’Write-Host “Test: [$test]” |
The variable $test is automatically expanded within the string.
To display object properties within an expanded string, use a sub-expression:
$process = Get-Process Chrome$process | ForEach-Object { Write-Host “Process: $($_.Name)”} |
This method ensures that the object properties are correctly expanded in the string.
Literal Strings
Literal strings, enclosed in single quotes, do not perform variable expansion. They display the string content exactly as it is. For example:
$test = ‘This is a test’Write-Host ‘This is a literal string: [$test]’ |
The variable $test remains unexpended within the literal string.
The -f Operator
PowerShell’s -f operator is a powerful tool for string formatting. It enables you to format strings with placeholders for variables, making the output more readable and structured. Here’s an example:
$user = (Get-ChildItem Env:\USERNAME). Value$date = Get-Date”Your user name is {0}, and the time is [{1:HH}:{1:mm}:{1:ss}]” -f $user, $date |
The -f operator simplifies string formatting, especially when dealing with complex output.
Converting to and from Strings
In PowerShell, you can easily convert objects to strings using the ToString() method or by casting them to [string]. Conversely, you can cast strings back to their original object types.
For example, to convert an integer to a string:
$number = 10$numberString = $number.ToString() |
To cast a string to an integer:
$number = [int]$numberString |
This flexibility allows you to work seamlessly with different data types.
Building Scriptblocks with Strings
Strings play a crucial role in building dynamic scriptblocks in PowerShell. You can use expanded strings to construct scriptblocks and then execute them using various cmdlets like Start-Job.
Here’s an example where we create a scriptblock to find a specific process:
$findProcess = ‘chrome’$expression = [scriptblock]::Create(“Get-Process $findProcess | Select-Object ProcessName, CPU, Path | Format-List”)Start-Job -Name “$findProcess`Process” -ScriptBlock $expression |
In this script, an expanded string constructs the scriptblock, allowing us to dynamically search for a process.
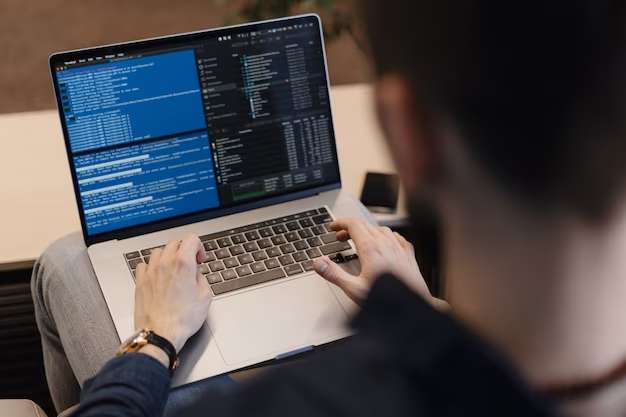
String Manipulation in PowerShell
In PowerShell, strings are versatile and can be manipulated in various ways. Here are some key techniques for string manipulation:
- Substring: Use the SubString method to extract a portion of a string based on its position and length. For example, $string.SubString(0, 5) would extract the first 5 characters of the string;
- LastIndexOf: The LastIndexOf method helps you find the position of the last occurrence of a specific character or substring within a string. It’s handy for extracting filenames or directory names from paths;
- Expanded Strings: Enclose strings in double quotes to create expanded strings. You can insert variable values directly into the string, making it a dynamic and informative way to display data;
- Literal Strings: Literal strings, enclosed in single quotes, are useful when you want to display the text exactly as it is, without variable substitution. These are often used for static text or paths;
- The -f Operator: PowerShell’s -f operator allows you to format strings with placeholders. You can replace placeholders with variables or values, providing structured output;
- Converting to and from Strings: You can convert other data types, such as integers or objects, to strings using the ToString method. Similarly, you can cast a variable to a string type;
- Building ScriptBlocks: You can use expanded strings to dynamically create script blocks. This is useful for constructing complex commands and executing them as jobs.
String manipulation is an essential skill in PowerShell, as it enables you to work with text data efficiently. These techniques will help you process, format, and present strings effectively in your scripts and automation tasks.
Video Guide
To finally answer all your questions, we have prepared a special video for you. Enjoy watching it!
Conclusion
PowerShell strings are versatile and offer numerous ways to manipulate, format, and integrate them into your scripts. Whether you’re extracting data from paths, formatting output, or building dynamic scriptblocks, a solid understanding of string manipulation is essential for mastering PowerShell.
By mastering these string manipulation techniques, you’ll be better equipped to tackle complex scripting tasks and unleash the full potential of PowerShell in your automation workflows.