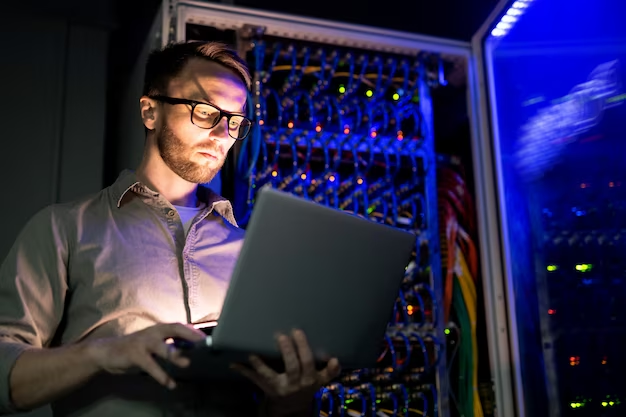
PowerShell Background Jobs: A Comprehensive Guide
In the realm of PowerShell, background jobs provide a powerful means to execute commands asynchronously, allowing you to manage tasks more efficiently. This comprehensive guide will walk you through the intricacies of background jobs in PowerShell, from their creation to monitoring, error handling, and cleanup.
Introduction to PowerShell Background Jobs
Background jobs are an essential feature of PowerShell, enabling you to run commands in parallel, monitor their progress, and collect results without blocking the main console. Whether you need to perform multiple tasks concurrently or execute long-running operations without interruption, PowerShell jobs have got you covered.
Why Use PowerShell Jobs?
PowerShell jobs offer a myriad of advantages, making them indispensable in various scenarios:
- Parallel Execution: You can run multiple jobs simultaneously, significantly improving task completion times;
- Efficient Resource Utilization: Jobs can be initiated as 64-bit or 32-bit processes, regardless of your session’s architecture, optimizing resource utilization;
- Cross-User Execution: Start jobs that run under different user contexts, enhancing security and flexibility.
In this guide, we’ll delve into the key aspects of PowerShell background jobs, equipping you with the knowledge to harness their full potential.
Creating PowerShell Jobs
The journey into the world of PowerShell jobs begins with the Start-Job cmdlet. This cmdlet allows you to initiate a background job, defining the task it should perform.
Let’s consider a simple example:
Start-Job -Name SleepProcess -ScriptBlock {Start-Sleep -Seconds 60; Get-Process} |
In this command, we start a job named “SleepProcess” that will wait for one minute (thanks to Start-Sleep) and then execute Get-Process.
Monitoring Running Jobs
Once you’ve launched a job, you’ll likely want to monitor its progress. To do so, use the Get-Job cmdlet:
Get-Job |
This command displays a list of all running jobs by default, making it easy to keep an eye on their status.
Collecting Information from Jobs
To gather information from running jobs, PowerShell provides the Receive-Job cmdlet. You can specify the job’s name or ID to retrieve its output. For instance:
Receive-Job -Id 1 -Keep |
The -Keep parameter allows you to store the job’s output for future reference. Additionally, PowerShell stores job information within child jobs, accessible through the ChildJobs property.
Error Handling in PowerShell Jobs
Dealing with errors in PowerShell jobs requires careful consideration, as job states might not always accurately reflect the actual outcome. There are two main scenarios to address when handling errors in jobs: when a job’s state is “Failed” and when it’s “Completed.”
Handling Failed Jobs
Consider the following example:
$failJob = Start-Job -Name FailJob -ScriptBlock {New-Item -Path ‘Z:\’ -Name ‘test’ -ItemType Directory -ErrorAction Stop} |
In this case, the job’s state appears as “Completed,” but it has indeed failed. To extract error information, you can access the JobStateInfo.Reason.Message property of the child job:
$failJob.ChildJobs[0].JobStateInfo.Reason.Message |
This approach retrieves the error message as a string.
Handling Completed Jobs
When a job is completed but contains errors, the error message can be found within the ChildJobs property. For instance:
$failJob = Start-Job -Name FailJob -ScriptBlock {New-Item -Path ‘Z:\’ -Name ‘test’ -ItemType Directory} |
In this scenario, the error information is stored in the Error property of the child job:
$failJob.ChildJobs[0].Error |
These methods help you effectively manage errors and troubleshoot issues within your PowerShell jobs.
Cleanup of PowerShell Jobs
Managing your jobs efficiently also involves proper cleanup to remove completed or unwanted jobs. The Remove-Job cmdlet serves this purpose, allowing you to delete specific jobs by name or ID or even all jobs at once:
# Remove a specific job by name or IDRemove-Job -Name MyJobRemove-Job -Id 1 # Remove all jobsGet-Job | Remove-Job |
By employing job cleanup practices, you can maintain a clutter-free PowerShell environment.
Putting It All Together: Running Multiple Jobs
One of the most compelling features of PowerShell jobs is the ability to execute multiple commands concurrently. In this example, we demonstrate how to initiate several jobs and monitor them using a While loop:
# Set the jobs variable to $true to enter the while loop$jobs = $true # Define the output folder path$outputFolder = ‘C:\PowerShell\part10\output’ # Create jobsStart-Job -Name SleepProcess -ScriptBlock {Start-Sleep -Seconds 60; Get-Process}Start-Job -Name FailJob -ScriptBlock {New-Item -Path ‘Z:\’ -Name ‘test’ -ItemType Directory -ErrorAction Stop}Start-Job -Name FailCompletedJob -ScriptBlock {New-Item -Path ‘Z:\’ -Name ‘test’ -ItemType Directory} # Check for and manage jobsWhile ($jobs) { $ourJobs = Get-Job # Iterate through jobs foreach ($jobObject in $ourJobs) { # Handle job states Switch ($jobObject.State) { # Running job {$_ -eq ‘Running’} { # Display job information Write-Host “Job: [$($jobObject.Name)] is still running…”`n Write-Host “Command: $($jobObject.Command)`n” } # Completed job {$_ -eq ‘Completed’} { # Create a job file with results # Handle errors if present # Remove the job } # Failed job {$_ -eq ‘Failed’} { # Create a job file with error information # Remove the job } } } # Wait for jobs and update the $jobs variable # as needed to continue or exit the loop Start-Sleep -Seconds 10} |
This script illustrates the simultaneous execution of multiple jobs, monitoring their progress, handling errors, and ensuring proper cleanup.
Best Practices for Using PowerShell Jobs
When working with PowerShell jobs, it’s essential to follow best practices to ensure efficiency and avoid potential issues. Here are some recommendations:
- Use Jobs Sparingly: While PowerShell jobs are powerful, avoid creating an excessive number of jobs simultaneously. Overloading your system with too many concurrent jobs can lead to performance issues;
- Error Handling: Implement robust error handling within your job scripts. Since jobs can run asynchronously, capturing and handling errors effectively is crucial. Use Try-Catch blocks to manage errors gracefully;
- Job Cleanup: Always remove completed or failed jobs using the Remove-Job cmdlet. This prevents unnecessary resource consumption and keeps your PowerShell environment tidy;
- Resource Considerations: Be mindful of the system resources required for running multiple jobs concurrently. Jobs consume memory and processing power, so plan accordingly, especially on shared systems;
- Monitor Job Progress: Use Get-Job and Receive-Job to monitor job progress and collect results. Regularly check the status of your jobs to ensure they are running as expected;
- Avoid Infinite Loops: Be cautious when using While loops to monitor jobs. Ensure that your loop’s exit condition is well-defined to prevent infinite loops that may impact system performance;
- Remote Jobs: When using remote jobs with Invoke-Command -AsJob, ensure that you have the necessary permissions and connectivity to the target remote machine. Verify that the remote session remains open until the job is completed;
- Testing and Debugging: Test your jobs thoroughly in a controlled environment before deploying them in a production setting. Use the PowerShell Integrated Scripting Environment (ISE) or other debugging tools to identify and resolve issues;
- Logging and Reporting: Consider implementing logging mechanisms to record job activities and results. This can be invaluable for troubleshooting and auditing purposes;
- Documentation: Document your job scripts, including their purpose, expected inputs, and outputs. Proper documentation makes it easier for you and your team to manage and maintain jobs.
By following these best practices, you can harness the full potential of PowerShell jobs while minimizing potential challenges and disruptions in your automation workflows.
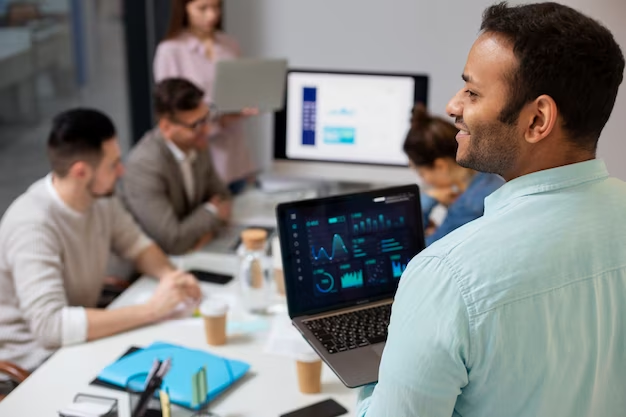
Video Guide
To finally answer all your questions, we have prepared a special video for you. Enjoy watching it!
Conclusion
PowerShell background jobs are an invaluable tool for streamlining your automation tasks, enhancing efficiency, and improving resource utilization. By mastering the creation, monitoring, error handling, and cleanup of jobs, you can unlock the full potential of PowerShell’s multitasking capabilities. Whether you’re a system administrator or a PowerShell enthusiast, incorporating jobs into your scripting arsenal will undoubtedly elevate your PowerShell proficiency.