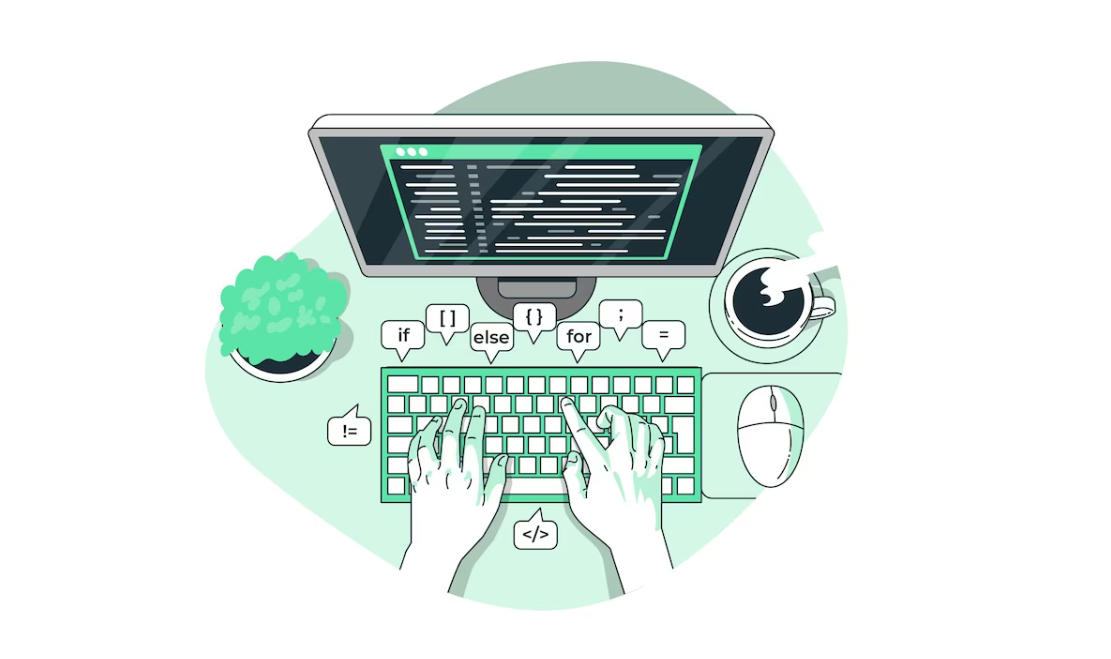
PowerShell Illustrated: Mastering Switch
PowerShell is a versatile and powerful scripting language that is essential for system administrators, IT professionals, and developers in the Windows ecosystem. It provides a robust set of tools for automating tasks, managing systems, and working with data. One of the fundamental constructs in PowerShell is the Switch statement, which allows you to make decisions based on the value of an expression. In this article, we will explore the Switch statement in PowerShell by using practical examples to illustrate its utility.
Enhancing Code Clarity with Switch Statements in PowerShell
Switch statements offer an efficient means of executing varying code based on distinct conditions, often proving more streamlined than employing multiple if/elseif statements.
$month = 3
if ($month -eq 1) { Write-Host "January" }
elseif ($month -eq 2) { Write-Host "February" }
elseif ($month -eq 3) { Write-Host "March" }
elseif ($month -eq 4) { Write-Host "April" }
elseif ($month -eq 5) { Write-Host "May" }
elseif ($month -eq 6) { Write-Host "June" }
elseif ($month -eq 7) { Write-Host "July" }
elseif ($month -eq 8) { Write-Host "August" }
elseif ($month -eq 9) { Write-Host "September" }
elseif ($month -eq 10) { Write-Host "October" }
elseif ($month -eq 11) { Write-Host "November" }
elseif ($month -eq 12) { Write-Host "December" }
else { Write-Host "Invalid month" }
# Instead we can write the above as
switch ($month) {
1 { Write-Host "January" }
2 { Write-Host "February" }
3 { Write-Host "March" }
4 { Write-Host "April" }
5 { Write-Host "May" }
6 { Write-Host "June" }
7 { Write-Host "July" }
8 { Write-Host "August" }
9 { Write-Host "September" }
10 { Write-Host "October" }
11 { Write-Host "November" }
12 { Write-Host "December" }
default { Write-Host "Invalid month" }
}
Result:
March
March
While the outcome remains unchanged, the code significantly improves in readability.
Wildcard Usage
Wildcards can be employed within the case condition:
# Using the -Wildcard parameter
$msg = "Error, the action failed"
switch -Wildcard ($msg) {
"Error*" { "Action error" }
"Warning*" { "Action warning" }
"Successful*" { "Action succesfull" }
}
## Or use it in the conditions
$msg = "Error, the action failed"
switch ($msg) {
{ $_ -like "Error*" } { "Action error" }
{ $_ -like "Warning*" } { "Action warning" }
{ $_ -like "Successful*" } { "Action succesfull" }
}
Result:
Action error
Action error
Both yield identical results, yet the latter example offers greater flexibility in choosing the operators you wish to employ.
Handling Multiple Conditions
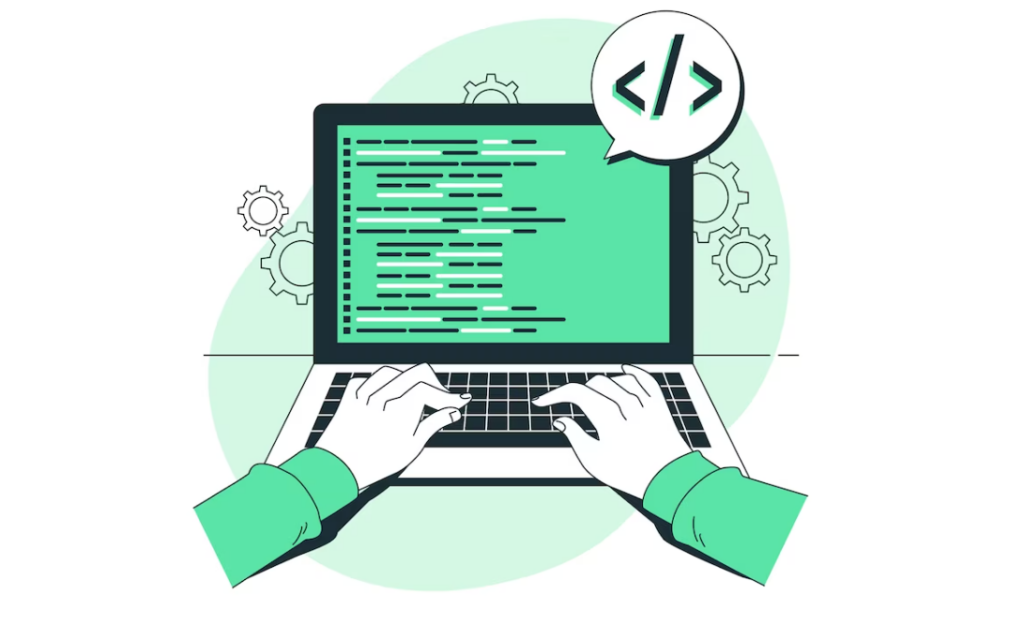
When employing multiple expressions within a switch statement, you can utilize the -and and -or operators.
switch ((Get-Date).Day) {
{ $_ -le 10 } { "Day of the month is lower than 10" }
{ $_ -gt 10 -and $_ -le 25 } { "Day of the month is between 10 and 25" }
{ $_ -gt 25 } { "Day of the month is greater than 25" }
}
Result:
Day of the month is between 10 and 25
Conclusion
PowerShell’s Switch statement is a powerful tool for improving scripting efficiency and code clarity. It simplifies complex decision-making, making it essential for Windows system administrators, IT professionals, and developers. Whether you’re converting months to text, handling wildcard patterns, or dealing with complex conditions, mastering the Switch statement streamlines scripting tasks, resulting in more effective and maintainable scripts.