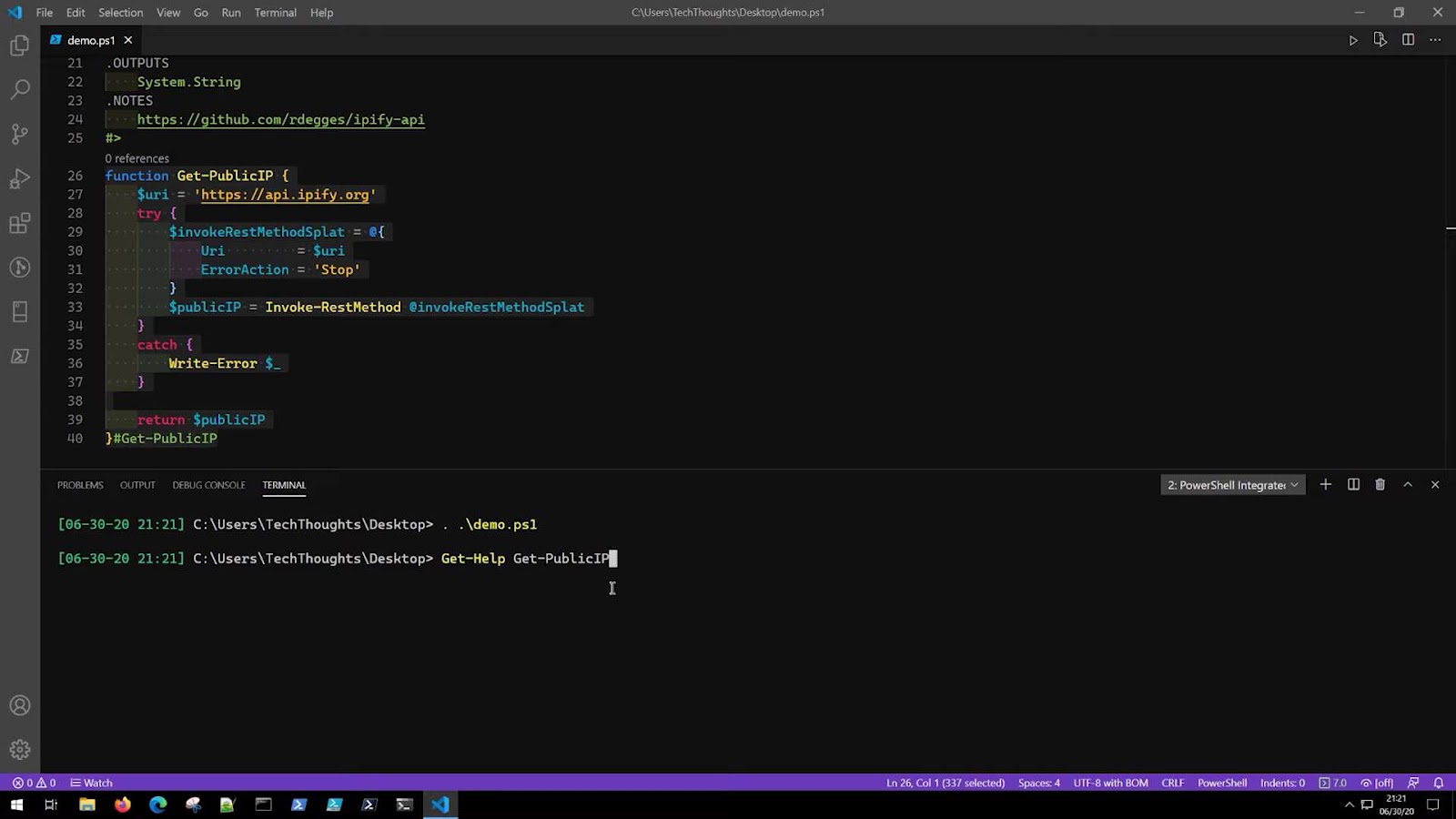
Unlocking the Potential of PowerShell’s Functions
In PowerShell, the use of functions is not obligatory, yet they prove invaluable when your code starts exhibiting repetitive patterns. Furthermore, when you embark on the creation of PowerShell modules, it is highly advisable to contemplate encapsulating your code within functions. This not only enhances the legibility of your code but also contributes to its overall organization.
Understanding Functions in Programming
In the vast realm of programming, a function serves as a fundamental building block. Think of it as a small machine within a larger factory, each designed to perform a specific task. To give an introduction, let’s delve into the concept of creating functions.
Crafting a Basic Function
In many programming environments, the keyword function initiates the creation of a function. Here’s a rudimentary illustration:
function displayHelloWorld() {
console.log("Hello World!");
}
displayHelloWorld();
Output:
Hello World!
Though this function might seem too elementary, it underscores the simplicity behind function creation. However, the true potential of functions is unleashed when they are provided with information to process, commonly known as ‘parameters’.
Unraveling the Power of Parameters
Parameters are akin to the dials and switches on machinery. They allow for customization of a function’s behavior based on varying inputs. Designing a function to accept parameters adds versatility to its utility.
Here’s a demonstration of how to create a function with parameters:
function displayCustomMessage(Message) {
console.log("Message: " + Message);
}
displayCustomMessage("Hello World!");
displayCustomMessage("Who is there?");
Output:
Message: Hello World!
Message: Who is there?
From the above illustration, it’s evident that parameters can be passed in multiple ways. Sometimes, they’re specified directly, and other times, they might be passed using their respective parameter names for clarity.
Enhancing Function Capabilities in PowerShell
In the realm of PowerShell scripting, enhancing a function’s features can provide greater control, flexibility, and more user-friendly experiences. One way to upscale a function’s potential is by incorporating the [CmdletBinding()] attribute. This attribute introduces a suite of advanced characteristics to the function, such as:
- Impact Confirmation: This allows users to receive a prompt, ensuring they’re aware of the consequences before executing a specific action. It acts as a safety check, especially for commands that might have high-impact outcomes;
- Help URI: An associated URI can be specified, directing users to detailed documentation or assistance related to the function. This fosters a better understanding of the function and its proper use;
- Support for Paging: For functions returning extensive outputs, paging support helps break down the output, making it easier to digest in segmented views;
- Should Process: It provides conditional processing capabilities. This can be beneficial when certain conditions must be met before proceeding with an action;
- Positional Binding: This ensures that parameters are accepted in a specific order, enhancing accuracy and predictability during function calls.
Consider the following illustrative example of a PowerShell function:
function Write-EnhancedMessage {
[CmdletBinding()]
param(
[Parameter(Mandatory = $true, Position = 0, HelpMessage = "Input the desired message")]
[string]$InputMessage
)
process {
Write-Host "Displayed Message: $InputMessage"
}
}
Write-EnhancedMessage "Greetings from the digital realm!"
Write-EnhancedMessage
The execution will produce:
Displayed Message: Greetings from the digital realm!
cmdlet Write-EnhancedMessage at command pipeline position 1
Provide values for the subsequent parameters:
InputMessage:
Write-EnhancedMessage: C:\path\script.ps1:15:1
Line |
15 | Write-EnhancedMessage
| ~~~~~~~~~~~~~~~~~~~~~~
| Unable to bind argument to 'InputMessage' due to it being a null or empty string.
This example also incorporates the process block, which is essential when managing the primary activities of a function, especially when handling pipeline inputs.
By understanding and implementing such enhancements, scripters can deliver more robust, user-friendly, and professional-grade functions in PowerShell.
Breaking Down Script Execution: The Roles of Begin, Process, and End
In PowerShell scripting, it is sometimes essential to structure a function for optimal flow and efficiency. This often involves categorizing operations into distinct segments for clarity and ease of management. Three prominent segments commonly seen in scripts are ‘Begin’, ‘Process’, and ‘End’.
1. The “Begin” Segment:
The ‘Begin’ block, as the name suggests, is the segment that initiates the script’s operations. It sets the stage for the function, preparing any necessary conditions before the primary action is executed. This segment is pivotal for:
- Validating inputs;
- Setting up prerequisites like initializing variables;
- Displaying preliminary messages or warnings;
- Checking conditions that may affect the rest of the script.
2. The “Process” Segment:
This is the heart of the function. It’s where the primary operation or task the function is designed for takes place. The ‘Process’ block is called for every input provided to the function, which makes it essential for:
- Handling the main logic of the function;
- Iterating through inputs and processing them;
- Generating outputs based on the function’s purpose.
3. The “End” Segment:
This is the closing act of the function. Once the main processing is done, the ‘End’ block can tidy up, provide summaries, or execute any final tasks that are deemed necessary. This segment is particularly useful for:
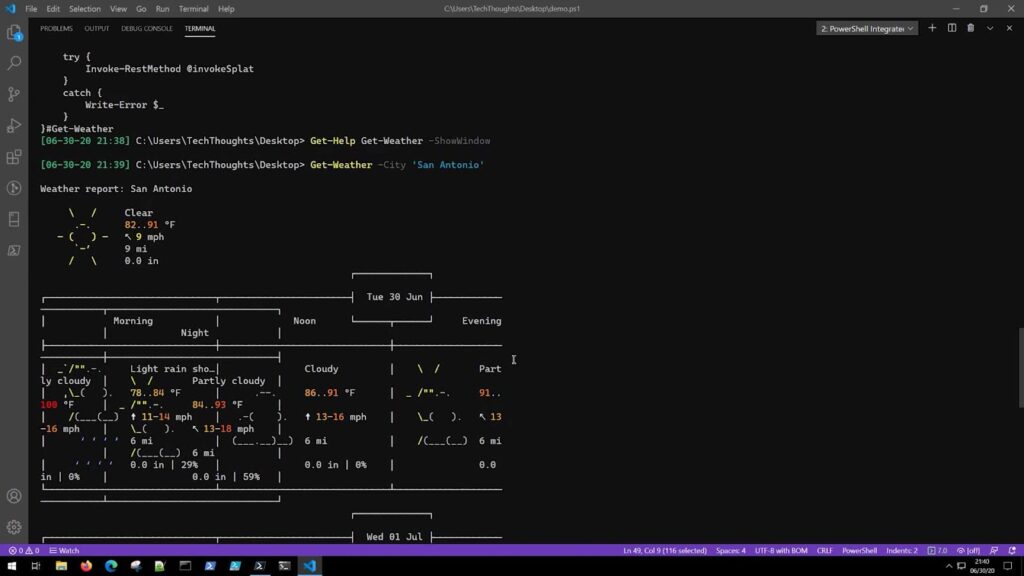
- Final reporting or output generation;
- Releasing resources or closing connections.
- Providing status updates or closing remarks.
Sample Script Explanation:
Consider the function writeMessage, which is structured with these three blocks:
function writeMessage {
[CmdLetBinding()]
param(
[Parameter(Mandatory = $true, Position = 1, HelpMessage = "The message to write")]
[string]$Message
)
begin {
Write-Verbose "Beginning of script"
if (($null -eq $Message) -or ($Message -eq "")) {
throw "Message cannot be empty";
}
}
process {
Write-Host "Message: $message"
}
end {
Write-Host "End of script"
}
}
- In the ‘Begin’ segment, there is a verbose message signaling the script’s commencement. Also, there’s a validation check to ensure the message isn’t empty;
- The ‘Process’ segment takes the validated message and displays it;
- Finally, the ‘End’ segment signifies the end of the script execution.
Upon running writeMessage “Hello World!” -Verbose, the function will display:
Beginning of script
Message: Hello World!
End of script
This structured approach ensures clarity, orderliness, and efficient management of function operations in PowerShell scripts.
Conclusion
In conclusion, PowerShell functions are an indispensable feature of this versatile scripting and automation language. They enable scriptwriters and administrators to modularize their code, improve code reusability, and enhance the maintainability of their scripts. Whether you are a seasoned PowerShell pro or just starting your journey, understanding how to create, use, and leverage functions can significantly boost your productivity and efficiency in managing Windows environments.